Spring BootとSpring Securityで安全なSPA認証機能を構築する方法
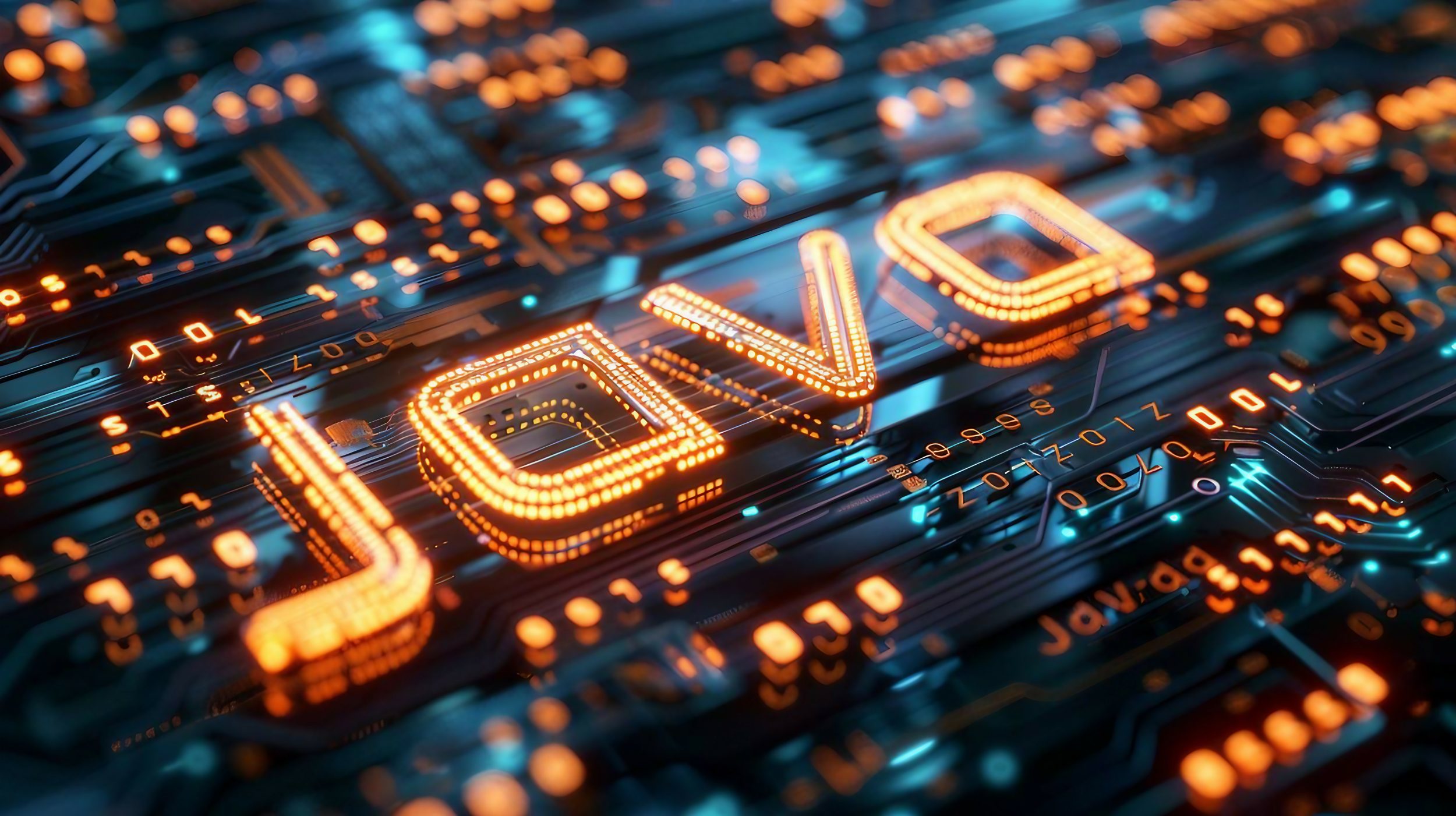
目次
Spring Boot × SPAアプリケーションにSpring Securityを導入する方法
Spring BootとSPA(Single Page Application)の組み合わせは、モダンなWebアプリケーション開発において非常に人気があります。
これにSpring Securityを導入することで、強力な認証機能を実現できます。
本記事では、Spring Securityの導入方法とその設定について詳しく解説します。
Spring Securityとは何か?
Spring Securityは、Spring Frameworkの一部として提供されるセキュリティフレームワークであり、認証と認可の機能を提供します。
これにより、アプリケーションに対する不正アクセスを防ぎ、安全な操作を保証します。
特に、Webアプリケーションではユーザー認証、権限管理、セッション管理などが重要です。
Spring Securityはこれらの機能を簡単に実装できるように設計されています。
Spring BootプロジェクトにSpring Securityを追加する手順
まず、Spring BootプロジェクトにSpring Securityを追加するには、依存関係を設定します。
`pom.xml`に以下の依存関係を追加します。
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
依存関係を追加したら、アプリケーションを再起動します。
これでSpring Securityが有効になり、デフォルトの設定が適用されます。
デフォルトでは、すべてのエンドポイントが認証を要求します。
SecurityConfig.javaの設定方法と解説
次に、認証と認可の設定を行うために`SecurityConfig.java`を作成します。
このクラスでは、特定のエンドポイントへのアクセスを制御し、カスタム認証プロバイダーを設定します。
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/public/**").permitAll() .anyRequest().authenticated() .and() .formLogin() .loginPage("/login") .permitAll() .and() .logout() .permitAll(); } }
この設定では、`/public`以下のURLは認証なしでアクセスでき、それ以外のURLは認証が必要です。
認証フィルタAuthorizeFilter.javaの実装方法
`AuthorizeFilter.java`は、特定の条件に基づいてリクエストをフィルタリングするために使用されます。
このフィルタを実装することで、カスタム認証ロジックを追加できます。
import java.io.IOException; import javax.servlet.FilterChain; import javax.servlet.ServletException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.security.core.context.SecurityContextHolder; import org.springframework.security.web.authentication.www.BasicAuthenticationFilter; public class AuthorizeFilter extends BasicAuthenticationFilter { public AuthorizeFilter() { super(authenticationManager()); } @Override protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException { String header = request.getHeader("Authorization"); if (header == null || !header.startsWith("Bearer ")) { chain.doFilter(request, response); return; } // Custom authentication logic here chain.doFilter(request, response); } }
このフィルタは、リクエストのヘッダーに含まれるトークンをチェックし、適切な認証処理を行います。
ユーザー詳細情報の設定: LoginUserDetailsとLoginUserDetailsService
`LoginUserDetails`と`LoginUserDetailsService`は、ユーザーの詳細情報を管理するためのクラスです。
これにより、ユーザーの認証情報を取得し、認証プロセスに組み込むことができます。
import org.springframework.security.core.GrantedAuthority; import org.springframework.security.core.userdetails.UserDetails; import java.util.Collection; public class LoginUserDetails implements UserDetails { private String username; private String password; private Collection<? extends GrantedAuthority> authorities; // Getters and setters @Override public Collection<? extends GrantedAuthority> getAuthorities() { return authorities; } @Override public String getPassword() { return password; } @Override public String getUsername() { return username; } @Override public boolean isAccountNonExpired() { return true; } @Override public boolean isAccountNonLocked() { return true; } @Override public boolean isCredentialsNonExpired() { return true; } @Override public boolean isEnabled() { return true; } }
`LoginUserDetailsService`では、ユーザー情報をデータベースから取得し、`LoginUserDetails`に設定します。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.core.userdetails.UsernameNotFoundException; import org.springframework.stereotype.Service; @Service public class LoginUserDetailsService implements UserDetailsService { @Autowired private UserRepository userRepository; @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { UserEntity user = userRepository.findByUsername(username); if (user == null) { throw new UsernameNotFoundException("User not found"); } return new LoginUserDetails(user); } }
これにより、ユーザーの認証情報を取得し、認証プロセスに組み込むことができます。
次に、Spring Securityを用いた認証機能の基礎と導入手順について解説します。
Spring Securityを用いた認証機能の基礎と導入手順
Spring Securityを用いた認証機能の実装は、Webアプリケーションにおいて非常に重要です。
ここでは、Spring Securityの基本概念から、具体的な導入手順までを解説します。
Spring Securityの基本概念
Spring Securityは、認証(authentication)と認可(authorization)を提供する強力なフレームワークです。
認証はユーザーの身元確認を行い、認可は特定のリソースへのアクセス権を管理します。
Spring Securityはこれらを簡単に実装できるため、多くの開発者に利用されています。
主な特徴として、カスタマイズ性が高く、既存のSpringアプリケーションに容易に統合できる点が挙げられます。
ユーザーエンティティ(UserEntity)の定義
ユーザーエンティティは、認証情報を保持するためのデータモデルです。
`UserEntity.java`を以下のように定義します。
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class UserEntity { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String username; private String password; private String roles; // Getters and setters }
このエンティティは、ユーザー名、パスワード、役割を格納します。
これにより、データベースからユーザー情報を取得しやすくなります。
ユーザーデータ管理: UserRepositoryの作成
ユーザーデータを管理するために、リポジトリを作成します。
`UserRepository.java`は以下の通りです。
import org.springframework.data.jpa.repository.JpaRepository; public interface UserRepository extends JpaRepository<UserEntity, Long> { UserEntity findByUsername(String username); }
このリポジトリは、ユーザー名でユーザー情報を検索するためのメソッドを提供します。
これにより、`LoginUserDetailsService`でユーザー情報を簡単に取得できます。
認証コントローラー(LoginController)の設定
次に、ユーザーのログインリクエストを処理するコントローラーを作成します。
`LoginController.java`は以下の通りです。
import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; @Controller public class LoginController { @GetMapping("/login") public String login() { return "login"; } @PostMapping("/login") public String loginSubmit() { // Handle login logic return "redirect:/"; } }
このコントローラーは、ログインページの表示とログイン処理を行います。
ユーザーフォーム(UserForm)の作成
最後に、ユーザー入力を受け取るためのフォームクラスを作成します。
`UserForm.java`は以下の通りです。
public class UserForm { private String username; private String password; // Getters and setters }
このフォームクラスは、ユーザー名とパスワードを受け取るためのフィールドを持ちます。
これにより、ログインページでユーザー入力を簡単に処理できます。
これで、Spring Securityを用いた認証機能の基礎と導入手順についての解説は完了です。
次に、Spring BootとSpring Securityで安全なSPA認証機能を構築する方法について解説します。
Spring BootとSpring Securityで安全なSPA認証機能を構築する方法
Spring BootとSpring Securityを使用して、SPA(Single Page Application)に安全な認証機能を導入する方法について解説します。
SPAとサーバーサイド認証の違い
SPAは、クライアントサイドで多くのロジックを処理するため、認証と認可の実装がサーバーサイドと異なります。
サーバーサイド認証では、すべてのリクエストがサーバーに送信され、サーバーが認証を行います。
一方、SPAでは、トークンベースの認証が一般的であり、クライアントサイドでトークンを保持し、リクエストごとにトークンを送信します。
項目 | SPA認証 | サーバーサイド認証 |
---|---|---|
認証方式 | トークンベース認証(例: JWT) | セッションベース認証 |
認証情報の保存場所 | クライアント側(ローカルストレージやCookie) | サーバー側(セッションストア) |
認証リクエストの頻度 | 初回のみ、以降はトークンを使用 | 各リクエストごとにセッション確認 |
認証情報の送信方法 | HTTPヘッダーにトークンを含める | CookieにセッションIDを含める |
セッション管理の負担 | クライアント側が管理 | サーバー側が管理 |
スケーラビリティ | 高い(トークンはステートレス) | 中程度(セッションストアのスケーリングが必要) |
セキュリティ上の考慮事項 | トークンの安全な保管、XSS対策 | セッションハイジャック対策、CSRF対策 |
初期セットアップの複雑さ | 中程度(トークンの生成と管理) | 低〜中(セッション管理と認証ロジックの実装) |
パフォーマンス | 高い(サーバー負荷が軽減) | 低〜中(セッション管理による負荷) |
アプリケーションの例 | React、Angular、Vue.jsアプリケーション | 伝統的なWebアプリケーション(JSP、Thymeleafなど) |
Spring Securityを用いたSPA認証のアーキテクチャ
Spring Securityを用いたSPA認証の基本アーキテクチャは、JWT(JSON Web Token)を使用します。
ユーザーがログインすると、サーバーはJWTを生成し、クライアントに返します。
クライアントはこのトークンをローカルストレージに保存し、APIリクエスト時にトークンをヘッダーに含めて送信します。
サーバーはトークンを検証し、認証を行います。
SecurityConfig.javaの具体的な設定方法
JWT認証を実装するために、`SecurityConfig.java`を以下のように設定します。
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .csrf().disable() .authorizeRequests() .antMatchers("/api/public/**").permitAll() .anyRequest().authenticated() .and() .addFilter(new JWTAuthenticationFilter(authenticationManager())) .addFilter(new JWTAuthorizationFilter(authenticationManager())); } }
この設定では、`/api/public`以下のエンドポイントは認証なしでアクセスでき、それ以外のエンドポイントはJWT認証が必要です。
認証フィルタ(AuthorizeFilter.java)の役割と実装
`AuthorizeFilter.java`は、JWTを検証し、認証情報を設定するために使用されます。
以下は、JWT検証フィルタの例です。
import java.io.IOException; import javax.servlet.FilterChain; import javax.servlet.ServletException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.security.core.context.SecurityContextHolder; import org.springframework.security.web.authentication.www.BasicAuthenticationFilter; import io.jsonwebtoken.Jwts; public class JWTAuthorizationFilter extends BasicAuthenticationFilter { public JWTAuthorizationFilter(AuthenticationManager authManager) { super(authManager); } @Override protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException { String header = request.getHeader("Authorization"); if (header == null || !header.startsWith("Bearer ")) { chain.doFilter(request, response); return; } String token = header.substring(7); String username = Jwts.parser() .setSigningKey("SecretKey") .parseClaimsJws(token) .getBody() .getSubject(); if (username != null) { SecurityContextHolder.getContext().setAuthentication( new UsernamePasswordAuthenticationToken(username, null, new ArrayList<>())); } chain.doFilter(request, response); } }
このフィルタは、リクエストの`Authorization`ヘッダーに含まれるJWTを解析し、ユーザー情報を設定します。
LoginUserDetailsとLoginUserDetailsServiceの詳細
`LoginUserDetails`と`LoginUserDetailsService`は、ユーザーの詳細情報を管理するために使用されます。
これにより、ユーザーの認証情報を取得し、認証プロセスに組み込むことができます。
import org.springframework.security.core.GrantedAuthority; import org.springframework.security.core.userdetails.UserDetails; import java.util.Collection; public class LoginUserDetails implements UserDetails { private String username; private String password; private Collection<? extends GrantedAuthority> authorities; // Getters and setters @Override public Collection<? extends GrantedAuthority> getAuthorities() { return authorities; } @Override public String getPassword() { return password; } @Override public String getUsername() { return username; } @Override public boolean isAccountNonExpired() { return true; } @Override public boolean isAccountNonLocked() { return true; } @Override public boolean isCredentialsNonExpired() { return true; } @Override public boolean isEnabled() { return true; } }
`LoginUserDetailsService`では、ユーザー情報をデータベースから取得し、`LoginUserDetails`に設定します。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework .security.core.userdetails.UsernameNotFoundException; import org.springframework.stereotype.Service; @Service public class LoginUserDetailsService implements UserDetailsService { @Autowired private UserRepository userRepository; @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { UserEntity user = userRepository.findByUsername(username); if (user == null) { throw new UsernameNotFoundException("User not found"); } return new LoginUserDetails(user); } }
これにより、ユーザーの認証情報を取得し、認証プロセスに組み込むことができます。
次に、Spring Securityの設定方法: SecurityConfig.javaの詳細解説に進みます。
Spring Securityの設定方法: SecurityConfig.javaの詳細解説
Spring Securityの設定は、アプリケーションのセキュリティを管理する上で非常に重要です。
ここでは、`SecurityConfig.java`の詳細な設定方法について解説します。
SecurityConfig.javaとは?
`SecurityConfig.java`は、Spring Securityの設定を管理するためのクラスです。
このクラスでは、HTTPリクエストの認証と認可の設定、カスタム認証プロバイダーの追加などを行います。
基本的な設定: HTTPリクエストの設定
基本的な設定では、特定のURLパターンに対するアクセス制御を行います。
以下は、基本的なHTTPリクエストの設定例です。
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers("/public/**").permitAll() .anyRequest().authenticated() .and() .formLogin() .loginPage("/login") .permitAll() .and() .logout() .permitAll(); } }
この設定では、`/public`以下のURLは認証なしでアクセスでき、それ以外のURLは認証が必要です。
認証マネージャーの設定方法
認証マネージャーは、ユーザーの認証情報を管理するためのコンポーネントです。
`SecurityConfig.java`で認証マネージャーを設定する方法を以下に示します。
import org.springframework.context.annotation.Bean; import org.springframework.security.authentication.AuthenticationManager; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; @Configuration public class SecurityConfig extends WebSecurityConfigurerAdapter { @Autowired private UserDetailsService userDetailsService; @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.userDetailsService(userDetailsService).passwordEncoder(new BCryptPasswordEncoder()); } @Bean @Override public AuthenticationManager authenticationManagerBean() throws Exception { return super.authenticationManagerBean(); } }
この設定では、`UserDetailsService`を使用してユーザー情報をロードし、BCryptパスワードエンコーダーを使用してパスワードをハッシュ化します。
カスタム認証フィルタの追加方法
カスタム認証フィルタを追加することで、特定の認証ロジックを実装できます。
以下は、カスタム認証フィルタの追加方法です。
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter; @Configuration @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .anyRequest().authenticated() .and() .addFilterBefore(new CustomAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class); } }
この設定では、`CustomAuthenticationFilter`を認証フィルタチェーンに追加しています。
設定のベストプラクティス
Spring Securityの設定におけるベストプラクティスとして、以下の点に注意します。
1. セキュリティ設定は可能な限り具体的に行う。
2. デフォルトの設定を利用しつつ、必要に応じてカスタマイズする。
3. ユーザー情報やパスワードは安全に管理する。
これにより、安全かつ効率的なセキュリティ設定が可能になります。
次に、AuthorizeFilter.javaを活用した認証フィルタリングの実装手順について解説します。
AuthorizeFilter.javaを活用した認証フィルタリングの実装手順
`AuthorizeFilter.java`を使用することで、特定の条件に基づいてリクエストをフィルタリングし、認証プロセスをカスタマイズできます。
ここでは、その具体的な実装手順を解説します。
AuthorizeFilter.javaの役割とは?
`AuthorizeFilter.java`は、HTTPリクエストをインターセプトし、特定の条件に基づいてフィルタリングを行います。
これにより、カスタム認証ロジックを実装できます。
このフィルタは、Spring Securityのフィルタチェーンに組み込まれ、リクエストの処理前に実行されます。
フィルタの基本的な実装手順
フィルタを実装するために、`BasicAuthenticationFilter`を継承し、`doFilterInternal`メソッドをオーバーライドします。
以下に基本的な実装例を示します。
import java.io.IOException; import javax.servlet.FilterChain; import javax.servlet.ServletException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.security.core.context.SecurityContextHolder; import org.springframework.security.web.authentication.www.BasicAuthenticationFilter; public class AuthorizeFilter extends BasicAuthenticationFilter { public AuthorizeFilter(AuthenticationManager authenticationManager) { super(authenticationManager); } @Override protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException { String header = request.getHeader("Authorization"); if (header == null || !header.startsWith("Bearer ")) { chain.doFilter(request, response); return; } // Custom authentication logic here chain.doFilter(request, response); } }
このフィルタは、リクエストの`Authorization`ヘッダーをチェックし、カスタム認証ロジックを実行します。
認証フィルタのカスタマイズ方法
認証フィルタをカスタマイズすることで、特定の認証ロジックを実装できます。
以下は、JWTを使用したカスタム認証フィルタの例です。
import java.io.IOException; import javax.servlet.FilterChain; import javax.servlet.ServletException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.security.core.context.SecurityContextHolder; import org.springframework.security.web.authentication.www.BasicAuthenticationFilter; import io.jsonwebtoken.Jwts; public class JWTAuthorizationFilter extends BasicAuthenticationFilter { public JWTAuthorizationFilter(AuthenticationManager authenticationManager) { super(authenticationManager); } @Override protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException { String header = request.getHeader("Authorization"); if (header == null || !header.startsWith("Bearer ")) { chain.doFilter(request, response); return; } String token = header.substring(7); String username = Jwts.parser() .setSigningKey("SecretKey") .parseClaimsJws(token) .getBody() .getSubject(); if (username != null) { SecurityContextHolder.getContext().setAuthentication( new UsernamePasswordAuthenticationToken(username, null, new ArrayList<>())); } chain.doFilter(request, response); } }
このフィルタは、JWTを解析し、ユーザー情報を設定します。
フィルタリングのベストプラクティス
フィルタリングのベストプラクティスとして、以下の点に注意します。
1. フィルタは可能な限り軽量に保つ。
2. カスタム認証ロジックは、簡潔で効率的に実装する。
3. フィルタの順序を適切に設定し、セキュリティ上のギャップを防ぐ。
トラブルシューティングとデバッグ方法
フィルタの実装中に問題が発生した場合、以下の方法でトラブルシューティングとデバッグを行います。
1. ログを利用して、フィルタの動作を追跡する。
2. ブレークポイントを設定して、フィルタの内部状態を確認する。
3. テストケースを作成して、フィルタの動作を検証する。
これにより、フィルタの動作を確認し、問題を特定することができます。
次に、Spring Securityでのユーザー認証について解説します。
Spring Securityでのユーザー認証: UserEntity、UserRepository、LoginUserDetailsの使い方
Spring Securityを用いたユーザー認証は、ユーザー情報の管理と認証プロセスの設定が重要です。
ここでは、UserEntity、UserRepository、LoginUserDetailsの使い方について解説します。
UserEntityの定義と役割
UserEntityは、ユーザーの認証情報を保持するエンティティクラスです。
このクラスは、ユーザー名、パスワード、および役割を含むフィールドを持ちます。
以下は、UserEntityの定義例です。
import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class UserEntity { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String username; private String password; private String roles; // Getters and setters }
このエンティティは、データベースからユーザー情報を取得し、認証プロセスに使用されます。
UserRepositoryの作成と利用方法
UserRepositoryは、ユーザー情報をデータベースから取得するためのリポジトリクラスです。
このクラスは、Spring Data JPAを使用して簡単に作成できます。
以下は、UserRepositoryの定義例です。
import org.springframework.data.jpa.repository.JpaRepository; public interface UserRepository extends JpaRepository<UserEntity, Long> { UserEntity findByUsername(String username); }
このリポジトリは、ユーザー名でユーザー情報を検索するためのメソッドを提供します。
これにより、認証プロセスで必要なユーザー情報を取得できます。
LoginUserDetailsの設定方法
LoginUserDetailsは、ユーザーの認証情報を保持するためのクラスです。
このクラスは、UserDetailsインターフェースを実装し、ユーザーの詳細情報を提供します。
以下は、LoginUserDetailsの定義例です。
import org.springframework.security.core.GrantedAuthority; import org.springframework.security.core.userdetails.UserDetails; import java.util.Collection; public class LoginUserDetails implements UserDetails { private String username; private String password; private Collection<? extends GrantedAuthority> authorities; // Getters and setters @Override public Collection<? extends GrantedAuthority> getAuthorities() { return authorities; } @Override public String getPassword() { return password; } @Override public String getUsername() { return username; } @Override public boolean isAccountNonExpired() { return true; } @Override public boolean isAccountNonLocked() { return true; } @Override public boolean isCredentialsNonExpired() { return true; } @Override public boolean isEnabled() { return true; } }
このクラスは、ユーザー名、パスワード、および権限情報を保持します。
LoginUserDetailsServiceの実装方法
LoginUserDetailsServiceは、ユーザー情報をデータベースから取得し、LoginUserDetailsに設定するためのサービスクラスです。
このクラスは、UserDetailsServiceインターフェースを実装します。
以下は、LoginUserDetailsServiceの定義例です。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.security.core.userdetails.UserDetails; import org.springframework.security.core.userdetails.UserDetailsService; import org.springframework.security.core.userdetails.UsernameNotFoundException; import org.springframework.stereotype.Service; @Service public class LoginUserDetailsService implements UserDetailsService { @Autowired private UserRepository userRepository; @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { UserEntity user = userRepository.findByUsername(username); if (user == null) { throw new UsernameNotFoundException("User not found"); } return new LoginUserDetails(user); } }
このクラスは、ユーザー情報を取得し、LoginUserDetailsに設定します。
全体の連携と動作確認
これらのクラスを組み合わせることで、Spring Securityを用いたユーザー認証が実現します。
認証プロセスは以下のように動作します。
1. ユーザーがログインリクエストを送信。
2. LoginUserDetailsServiceがUserRepositoryを使用してユーザー情報を取得。
3. LoginUserDetailsにユーザー情報を設定。
4. SecurityConfigが認証プロセスを管理。
これにより、安全で効率的なユーザー認証が実現します。
次に、各設定の動作確認を行い、必要に応じて調整を行います。