Javaのポリモフィズムとは何か?その基本概念と理解のために
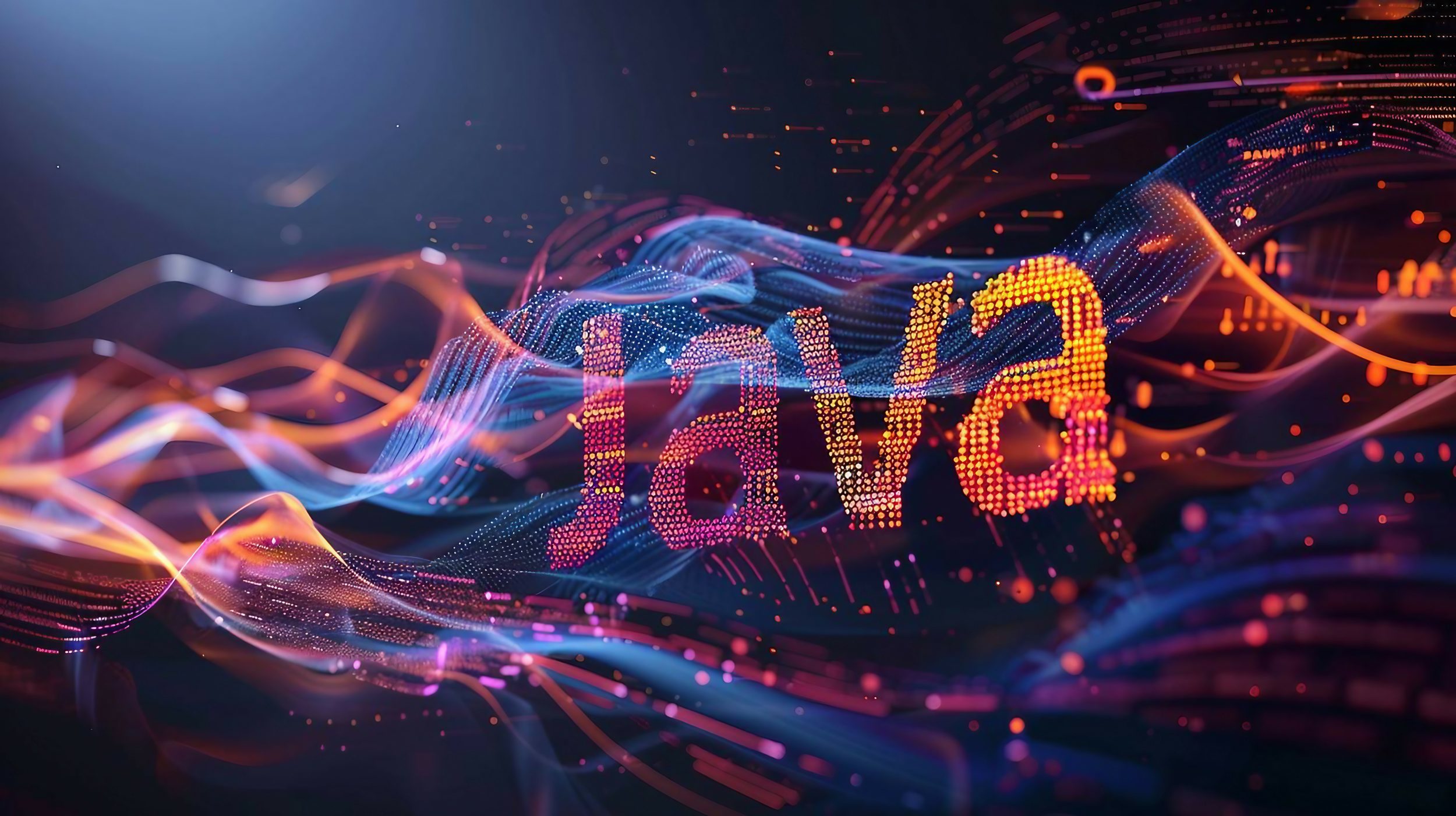
目次
Javaのポリモフィズムとは何か?その基本概念と理解のために
Javaのポリモフィズム(多態性)は、オブジェクト指向プログラミングの重要な概念の一つで、同じインターフェースを持つ複数のオブジェクトが、異なる方法で振る舞うことを可能にします。
ポリモフィズムにより、コードの再利用性が高まり、柔軟で拡張性のあるプログラムを作成することができます。
例えば、動物クラスを基底クラスとして、犬や猫などの派生クラスを作成する場合、各派生クラスは基底クラスのメソッドを独自に実装することができます。
これにより、同じメソッド呼び出しが異なる結果を生むことが可能となります。
class Animal { void sound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { void sound() { System.out.println("Dog barks"); } } class Cat extends Animal { void sound() { System.out.println("Cat meows"); } } public class TestPolymorphism { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.sound(); // Dog barks myCat.sound(); // Cat meows } }
上記の例では、`Animal`クラスが基底クラスであり、`Dog`と`Cat`クラスがその派生クラスです。
`TestPolymorphism`クラスの`main`メソッドで、`Animal`型の変数を使用して`Dog`および`Cat`オブジェクトを作成し、それぞれの`sound`メソッドを呼び出しています。
ポリモフィズムによって、同じ`sound`メソッドが異なる動作をすることが確認できます。
ポリモフィズムの定義とその重要性
ポリモフィズム(多態性)とは、プログラムのコンテキストに応じて異なる方法で振る舞うことができる能力を指します。
これは、オブジェクト指向プログラミング(OOP)の四大原則の一つであり、他の三つはカプセル化、継承、そして抽象化です。
ポリモフィズムは、特定のクラスに依存せずにプログラムを柔軟にし、コードの再利用性とメンテナンス性を向上させる重要な役割を果たします。
これにより、異なるクラスが同じインターフェースを共有し、異なる方法で動作することが可能になります。
interface Shape { void draw(); } class Circle implements Shape { public void draw() { System.out.println("Drawing a Circle"); } } class Rectangle implements Shape { public void draw() { System.out.println("Drawing a Rectangle"); } } public class TestPolymorphism { public static void main(String[] args) { Shape myCircle = new Circle(); Shape myRectangle = new Rectangle(); myCircle.draw(); // Drawing a Circle myRectangle.draw(); // Drawing a Rectangle } }
上記の例では、`Shape`インターフェースが定義され、それを実装する`Circle`と`Rectangle`クラスがあります。
`TestPolymorphism`クラスの`main`メソッドで、`Shape`型の変数を使用して`Circle`および`Rectangle`オブジェクトを作成し、それぞれの`draw`メソッドを呼び出しています。
このように、ポリモフィズムにより、異なるクラスが同じインターフェースを実装し、それぞれ異なる動作をすることが可能になります。
ポリモフィズムがJavaでどのように実現されるか
Javaでは、ポリモフィズムは主に継承とインターフェースを通じて実現されます。
クラスが他のクラスを継承する場合、サブクラスはスーパークラスのメソッドをオーバーライドして独自の実装を提供できます。
また、インターフェースを実装するクラスは、インターフェースで宣言されたメソッドをすべて実装する必要があります。
これにより、異なるクラスが同じメソッド名を持ち、異なる振る舞いを実現することができます。
interface Vehicle { void start(); } class Car implements Vehicle { public void start() { System.out.println("Car starts"); } } class Motorcycle implements Vehicle { public void start() { System.out.println("Motorcycle starts"); } } public class TestVehicle { public static void main(String[] args) { Vehicle myCar = new Car(); Vehicle myMotorcycle = new Motorcycle(); myCar.start(); // Car starts myMotorcycle.start(); // Motorcycle starts } }
この例では、`Vehicle`インターフェースを実装する`Car`および`Motorcycle`クラスがあります。
`TestVehicle`クラスでは、`Vehicle`型の変数を使用して、車とオートバイの`start`メソッドを呼び出しています。
ポリモフィズムにより、同じ`start`メソッド呼び出しが異なる動作をすることが可能になります。
ポリモフィズムの歴史的背景とその進化
ポリモフィズムの概念は、オブジェクト指向プログラミング(OOP)の誕生とともに発展しました。
OOPの基礎は1960年代に登場し、SmalltalkやSimulaといった初期のOOP言語によって形作られました。
Javaは1990年代に登場し、OOPの原則を採用しつつ、C++の複雑さを排除することを目指しました。
ポリモフィズムは、ソフトウェア設計の柔軟性を高めるために重要な役割を果たし、現代の多くのプログラミング言語において標準的な機能となっています。
abstract class Animal { abstract void makeSound(); } class Dog extends Animal { void makeSound() { System.out.println("Dog barks"); } } class Cat extends Animal { void makeSound() { System.out.println("Cat meows"); } } public class TestAnimal { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.makeSound(); // Dog barks myCat.makeSound(); // Cat meows } }
この例では、抽象クラス`Animal`を使用してポリモフィズムを実現しています。
`Dog`と`Cat`クラスが`Animal`クラスを継承し、それぞれ`makeSound`メソッドを実装しています。
`TestAnimal`クラスでは、`Animal`型の変数を使用して、動物のサウンドを動的に変更しています。
ポリモフィズムを理解するための基本的な例
ポリモフィズムを理解するためには、簡単な例から始めるのが有効です。
例えば、動物の鳴き声をシミュレートするプログラムを考えてみましょう。
基本クラス`Animal`に`sound`メソッドを定義し、派生クラス`Dog`と`Cat`がそれぞれ異なる実装を持つ場合、ポリモフィズムにより、同じ`sound`メソッド呼び出しが異なる動作をすることが確認できます。
class Animal { void sound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { void sound() { System.out.println("Dog barks"); } } class Cat extends Animal { void sound() { System.out.println("Cat meows"); } } public class TestPolymorphism { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.sound(); // Dog barks myCat.sound(); // Cat meows } }
この例では、`Animal`クラスが基底クラスであり、`Dog`と`Cat`クラスがその派生クラスです。
ポリモフィズムにより、同じ`sound`メソッド呼び出しが異なる動作をすることが確認できます。
ポリモフィズムの利点とその応用
範囲ポリモフィズムには多くの利点があり、さまざまな応用範囲があります。
主な利点として、コードの再利用性、保守性、拡張性が挙げられます。
例えば、GUIフレームワークやデザインパターン、ゲーム開発など、多くの分野でポリモフィズムが活用されています。
これにより、開発効率が向上し、複雑なソフトウェアシステムの管理が容易になります。
interface Notification { void send(String message); } class EmailNotification implements Notification { public void send(String message) { System.out.println("Sending email: " + message); } } class SMSNotification implements Notification { public void send(String message) { System.out.println("Sending SMS: " + message); } } public class TestNotification { public static void main(String[] args) { Notification notification = new EmailNotification(); notification.send("Hello via Email"); // Sending email: Hello via Email notification = new SMSNotification(); notification.send("Hello via SMS"); // Sending SMS: Hello via SMS } }
この例では、`Notification`インターフェースを使用して、`EmailNotification`と`SMSNotification`クラスが定義されています。
`TestNotification`クラスでは、`Notification`型の変数を使用して通知方法を変更しています。
ポリモフィズムにより、異なる通知方法を簡単に追加・変更でき、コードの柔軟性が向上しています。
ポリモフィズムが実現する交換可能な機能とは何か?
ポリモフィズムの最大の利点の一つは、交換可能な機能を実現することです。
交換可能な機能とは、あるクラスのオブジェクトを別のクラスのオブジェクトに置き換えても、そのプログラムの基本的な動作が変わらないことを指します。
これは、インターフェースや基底クラスを使用して実現されます。
これにより、特定の機能を持つクラスを後から簡単に差し替えることができ、プログラムの柔軟性と拡張性が向上します。
interface Payment { void pay(int amount); } class CreditCardPayment implements Payment { public void pay(int amount) { System.out.println("Paid " + amount + " using Credit Card"); } } class PayPalPayment implements Payment { public void pay(int amount) { System.out.println("Paid " + amount + " using PayPal"); } } public class TestPayment { public static void main(String[] args) { Payment payment = new CreditCardPayment(); payment.pay(100); // Paid 100 using Credit Card payment = new PayPalPayment(); payment.pay(200); // Paid 200 using PayPal } }
この例では、`Payment`インターフェースが定義され、それを実装する`CreditCardPayment`と`PayPalPayment`クラスがあります。
`TestPayment`クラスの`main`メソッドで、`Payment`型の変数を使用して支払い方法を変更しています。
ポリモフィズムにより、`CreditCardPayment`から`PayPalPayment`に簡単に切り替えることができ、コードの柔軟性が向上しています。
交換可能な機能の概念とその必要性
交換可能な機能は、プログラムの柔軟性を高め、変更に強い設計を可能にします。
例えば、異なる支払い方法をサポートするアプリケーションを考えた場合、ポリモフィズムを使用することで、新しい支払い方法を追加する際に既存のコードを変更する必要がなくなります。
これにより、保守性が向上し、開発コストが削減されます。
interface Notification { void send(String message); } class EmailNotification implements Notification { public void send(String message) { System.out.println("Sending email: " + message); } } class SMSNotification implements Notification { public void send(String message) { System.out.println("Sending SMS: " + message); } } public class TestNotification { public static void main(String[] args) { Notification notification = new EmailNotification(); notification.send("Hello via Email"); // Sending email: Hello via Email notification = new SMSNotification(); notification.send("Hello via SMS"); // Sending SMS: Hello via SMS } }
上記の例では、`Notification`インターフェースを使用して、`EmailNotification`と`SMSNotification`クラスが定義されています。
`TestNotification`クラスでは、`Notification`型の変数を使用して、通知方法を変更しています。
ポリモフィズムにより、通知方法を簡単に変更できるため、コードの再利用性と柔軟性が向上しています。
交換可能な機能の実装例
交換可能な機能を実装するためには、インターフェースや抽象クラスを使用して、同じメソッドシグネチャを持つ複数のクラスを定義します。
これにより、プログラムの特定の部分を簡単に置き換えることができます。
以下は、異なる支払い方法を実装する例です。
interface Payment { void pay(int amount); } class BitcoinPayment implements Payment { public void pay(int amount) { System.out.println("Paid " + amount + " using Bitcoin"); } } public class TestPayment { public static void main(String[] args) { Payment payment = new BitcoinPayment(); payment.pay(300); // Paid 300 using Bitcoin } }
この例では、`Payment`インターフェースを使用して、新しい支払い方法`BitcoinPayment`クラスが追加されています。
`TestPayment`クラスでは、`Payment`型の変数を使用して支払い方法を変更しています。
ポリモフィズムにより、新しい支払い方法を簡単に追加でき、コードの柔軟性が向上しています。
交換可能な機能の利点とその影響
交換可能な機能の利点には、コードの再利用性、保守性、拡張性の向上が含まれます。
これにより、新しい機能を追加する際に既存のコードを変更する必要がなくなり、開発効率が向上します。
また、異なる実装を簡単に切り替えることができるため、プログラムの柔軟性が向上し、変更に強い設計が可能となります。
interface Logger { void log(String message); } class ConsoleLogger implements Logger { public void log(String message) { System.out.println("Console log: " + message); } } class FileLogger implements Logger { public void log(String message) { System.out.println("File log: " + message); } } public class TestLogger { public static void main(String[] args) { Logger logger = new ConsoleLogger(); logger.log("This is a console log."); // Console log: This is a console log. logger = new FileLogger(); logger.log("This is a file log."); // File log: This is a file log. } }
この例では、`Logger`インターフェースを使用して、`ConsoleLogger`と`FileLogger`クラスが定義されています。
`TestLogger`クラスでは、`Logger`型の変数を使用してログの出力方法を変更しています。
ポリモフィズムにより、異なるログの出力方法を簡単に追加・変更でき、コードの柔軟性が向上しています。
交換可能な機能の実装におけるベストプラクティス
交換可能な機能を実装する際のベストプラクティスとしては、インターフェースを適切に設計し、実装クラスが単一の責任を持つようにすることが重要です。
また、依存性注入(DI)を使用して、オブジェクトの依存関係を管理しやすくすることも推奨されます。
これにより、コードのテストが容易になり、保守性が向上します。
interface Payment { void pay(int amount); } class CreditCardPayment implements Payment { public void pay(int amount) { System.out.println("Paid " + amount + " using Credit Card"); } } class PaymentService { private Payment payment; public PaymentService(Payment payment) { this.payment = payment; } public void processPayment(int amount) { payment.pay(amount); } } public class TestPaymentService { public static void main(String[] args) { Payment payment = new CreditCardPayment(); PaymentService service = new PaymentService(payment); service.processPayment(100); // Paid 100 using Credit Card } }
この例では、`Payment`インターフェースを使用して`CreditCardPayment`クラスが定義されています。
`PaymentService`クラスでは、依存性注入を使用して`Payment`オブジェクトを受け取り、`processPayment`メソッドで支払いを処理します。
ポリモフィズムにより、支払い方法を簡単に変更でき、コードの柔軟性が向上しています。
Javaでポリモフィズムをプログラムで実現する方法
Javaでポリモフィズムを実現するためには、基本的にインターフェースまたは継承を使用します。
これにより、異なるクラスが同じメソッドを実装し、実行時に動的に振る舞いを変更することができます。
インターフェースを使用する場合、複数のクラスが同じインターフェースを実装することで、同じメソッドシグネチャを持つ異なる動作を実現できます。
interface Vehicle { void start(); } class Car implements Vehicle { public void start() { System.out.println("Car starts"); } } class Motorcycle implements Vehicle { public void start() { System.out.println("Motorcycle starts"); } } public class TestVehicle { public static void main(String[] args) { Vehicle myCar = new Car(); Vehicle myMotorcycle = new Motorcycle(); myCar.start(); // Car starts myMotorcycle.start(); // Motorcycle starts } }
この例では、`Vehicle`インターフェースを使用して、`Car`および`Motorcycle`クラスが定義されています。
`TestVehicle`クラスでは、`Vehicle`型の変数を使用して、車とオートバイを動的に切り替えています。
ポリモフィズムにより、`start`メソッドが異なる動作をすることが確認できます。
インターフェースと継承を使ったポリモフィズムの実装方法
インターフェースと継承を使用することで、Javaでポリモフィズムを簡単に実装できます。
インターフェースを使用すると、クラスが同じメソッドシグネチャを持ち、異なる動作を実装することが可能です。
継承を使用すると、基底クラスのメソッドをオーバーライドして異なる動作を実装することができます。
abstract class Animal { abstract void makeSound(); } class Dog extends Animal { void makeSound() { System.out.println("Dog barks"); } } class Cat extends Animal { void makeSound() { System.out.println("Cat meows"); } } public class TestAnimal { public static void main(String[] args) { Animal myDog = new Dog(); Animal myCat = new Cat(); myDog.makeSound(); // Dog barks myCat.makeSound(); // Cat meows } }
上記の例では、抽象クラス`Animal`が定義され、`Dog`および`Cat`クラスがそれを継承しています。
`TestAnimal`クラスでは、`Animal`型の変数を使用して、動物のサウンドを動的に変更しています。
ポリモフィズムにより、`makeSound`メソッドが異なる動作をすることが確認できます。
継承とインターフェースの違いと使い分け
継承とインターフェースの主な違いは、継承はクラス間の「is-a」関係を表し、インターフェースは「can-do」関係を表します。
継承を使用すると、サブクラスはスーパークラスのすべてのメソッドとフィールドを継承しますが、インターフェースを実装するクラスは、インターフェースで定義されたメソッドのみを実装します。
interface Flyable { void fly(); } class Bird implements Flyable { public void fly() { System.out.println("Bird flies"); } } class Airplane implements Flyable { public void fly() { System.out.println("Airplane flies"); } } public class TestFlyable { public static void main(String[] args) { Flyable flyable = new Bird(); flyable.fly(); // Bird flies flyable = new Airplane(); flyable.fly(); // Airplane flies } }
この例では、`Flyable`インターフェースを実装する`Bird`および`Airplane`クラスが定義されています。
`TestFlyable`クラスでは、`Flyable`型の変数を使用して、飛行するオブジェクトを動的に切り替えています。
ポリモフィズムにより、異なるオブジェクトが同じメソッドを実装し、異なる動作をすることが確認できます。
インターフェースを使ったプログラムの利点
インターフェースを使用することで、Javaプログラムの柔軟性が向上します。
異なるクラスが同じインターフェースを実装することで、コードの再利用性が高まり、変更に強い設計が可能となります。
また、インターフェースを使用することで、依存性の低いコードを書くことができ、テストが容易になります。
interface Printer { void print(String document); } class InkjetPrinter implements Printer { public void print(String document) { System.out.println("Printing with Inkjet: " + document); } } class LaserPrinter implements Printer { public void print(String document) { System.out.println("Printing with Laser: " + document); } } public class TestPrinter { public static void main(String[] args) { Printer printer = new InkjetPrinter(); printer.print("Document 1"); // Printing with Inkjet: Document 1 printer = new LaserPrinter(); printer.print("Document 2"); // Printing with Laser: Document 2 } }
この例では、`Printer`インターフェースを使用して、`InkjetPrinter`および`LaserPrinter`クラスが定義されています。
`TestPrinter`クラスでは、`Printer`型の変数を使用して、プリンタを動的に切り替えています。
ポリモフィズムにより、異なるプリンタが同じメソッドを実装し、異なる動作をすることが確認できます。
継承を使ったプログラムの利点
継承を使用することで、コードの再利用性が向上し、同じコードを複数のクラスで共有することができます。
これにより、重複するコードを減らし、プログラムの保守性が向上します。
また、継承を使用することで、サブクラスはスーパークラスのメソッドをオーバーライドして独自の実装を提供できます。
class Employee { String name; int age; void work() { System.out.println(name + " is working"); } } class Manager extends Employee { void work() { System.out.println(name + " is managing the team"); } } public class TestEmployee { public static void main(String[] args) { Employee employee = new Employee(); employee.name = "John"; employee.work(); // John is working Manager manager = new Manager(); manager.name = "Jane"; manager.work(); // Jane is managing the team } }
この例では、`Employee`クラスが定義され、そのサブクラスとして`Manager`クラスが継承されています。
`TestEmployee`クラスでは、`Employee`型の変数を使用して、従業員とマネージャーの`work`メソッドを呼び出しています。
ポリモフィズムにより、`work`メソッドが異なる動作をすることが確認できます。
ポリモフィズムを使ったコードのテスト方法
ポリモフィズムを使ったコードのテストには、インターフェースや基底クラスを使用して、異なる実装クラスをテストします。
これにより、コードの動作を確認しやすくなり、バグの検出が容易になります。
JUnitなどのテストフレームワークを使用することで、テストの自動化も可能です。
interface Calculator { int calculate(int a, int b); } class Addition implements Calculator { public int calculate(int a, int b) { return a + b; } } class Subtraction implements Calculator { public int calculate(int a, int b) { return a - b; } } public class TestCalculator { public static void main(String[] args) { Calculator calc = new Addition(); System.out.println("Addition: " + calc.calculate(5, 3)); // Addition: 8 calc = new Subtraction(); System.out.println("Subtraction: " + calc.calculate(5, 3)); // Subtraction: 2 } }
この例では、`Calculator`インターフェースを使用して、`Addition`および`Subtraction`クラスが定義されています。
`TestCalculator`クラスでは、`Calculator`型の変数を使用して、計算方法を動的に切り替えています。
ポリモフィズムにより、異なる計算方法を簡単にテストでき、コードの柔軟性が向上しています。
ポリモフィズムを利用しない場合のコード例とその問題点
ポリモフィズムを利用しない場合、コードは一般的に冗長で、拡張性や保守性が低くなります。
異なる動作を実装するために、複数の条件分岐(`if`や`switch`文)を使用することが多く、その結果、コードが複雑になりやすいです。
以下は、ポリモフィズムを使用しない例です。
class PaymentProcessor { void processPayment(String paymentType, int amount) { if (paymentType.equals("CreditCard")) { System.out.println("Paid " + amount + " using Credit Card"); } else if (paymentType.equals("PayPal")) { System.out.println("Paid " + amount + " using PayPal"); } else { System.out.println("Invalid payment type"); } } } public class TestPaymentProcessor { public static void main(String[] args) { PaymentProcessor processor = new PaymentProcessor(); processor.processPayment("CreditCard", 100); // Paid 100 using Credit Card processor.processPayment("PayPal", 200); // Paid 200 using PayPal } }
この例では、`PaymentProcessor`クラスが特定の支払い方法を処理するために`if-else`文を使用しています。
新しい支払い方法を追加する場合は、このクラスを変更する必要があり、コードの保守性が低くなります。
また、複雑な条件分岐が増えると、バグの原因にもなります。
条件分岐を使ったプログラムの問題点
条件分岐を使ったプログラムは、以下のような問題点があります:
1. 保守性の低下:新しい機能を追加する際に、既存のコードを変更する必要があるため、コードの保守が難しくなります。
2. 拡張性の欠如:新しい種類のオブジェクトを追加するたびに、条件分岐のコードを修正しなければならないため、プログラムの拡張性が低くなります。
3. コードの可読性の低下:複雑な条件分岐が増えると、コードの可読性が低下し、理解しづらくなります。
4. バグの増加リスク:条件分岐が多くなると、バグが発生する可能性が高くなります。
class Shape { void draw(String shapeType) { if (shapeType.equals("Circle")) { System.out.println("Drawing a Circle"); } else if (shapeType.equals("Rectangle")) { System.out.println("Drawing a Rectangle"); } else { System.out.println("Invalid shape type"); } } } public class TestShape { public static void main(String[] args) { Shape shape = new Shape(); shape.draw("Circle"); // Drawing a Circle shape.draw("Rectangle"); // Drawing a Rectangle } }
この例では、`Shape`クラスが特定の形状を描画するために`if-else`文を使用しています。
新しい形状を追加する場合は、このクラスを変更する必要があり、コードの保守性と拡張性が低くなります。
ポリモフィズムを利用しない場合のメンテナンスの難しさ
ポリモフィズムを利用しない場合、メンテナンスは非常に困難になります。
特に、大規模なシステムでは、条件分岐の数が増えると、バグの修正や新機能の追加がますます難しくなります。
また、同じロジックが複数箇所に分散するため、一箇所の変更が他の箇所にどのように影響するかを理解するのが難しくなります。
class VehicleProcessor { void processVehicle(String vehicleType) { if (vehicleType.equals("Car")) { System.out.println("Processing a Car"); } else if (vehicleType.equals("Motorcycle")) { System.out.println("Processing a Motorcycle"); } else { System.out.println("Unknown vehicle type"); } } } public class TestVehicleProcessor { public static void main(String[] args) { VehicleProcessor processor = new VehicleProcessor(); processor.processVehicle("Car"); // Processing a Car processor.processVehicle("Motorcycle"); // Processing a Motorcycle } }
この例では、`VehicleProcessor`クラスが特定の車両タイプを処理するために`if-else`文を使用しています。
新しい車両タイプを追加する場合は、このクラスを変更する必要があり、メンテナンスが困難になります。
ポリモフィズムを利用しない場合のコードの冗長性
ポリモフィズムを利用しないコードは、冗長になりやすいです。
例えば、異なるタイプのオブジェクトに対して同じ操作を行う場合、同じようなコードを何度も書かなければならなくなります。
これにより、コードが冗長になり、保守が難しくなります。
class DocumentProcessor { void processDocument(String documentType) { if (documentType.equals("Word")) { System.out.println("Processing Word document"); } else if (documentType.equals("PDF")) { System.out.println("Processing PDF document"); } else { System.out.println("Unknown document type"); } } } public class TestDocumentProcessor { public static void main(String[] args) { DocumentProcessor processor = new DocumentProcessor(); processor.processDocument("Word"); // Processing Word document processor.processDocument("PDF"); // Processing PDF document } }
この例では、`DocumentProcessor`クラスが特定のドキュメントタイプを処理するために`if-else`文を使用しています。
新しいドキュメントタイプを追加する場合は、このクラスを変更する必要があり、コードが冗長になります。
ポリモフィズムを利用しない場合のバグのリスク
ポリモフィズムを利用しない場合、バグが発生するリスクが高まります。
特に、条件分岐が多くなると、誤った条件やタイプミスによりバグが発生しやすくなります。
また、新しい機能を追加する際に、既存のコードに影響を与える可能性が高くなります。
class AnimalProcessor { void processAnimal(String animalType) { if (animalType.equals("Dog")) { System.out.println("Processing a Dog"); } else if (animalType.equals("Cat")) { System.out.println("Processing a Cat"); } else { System.out.println("Unknown animal type"); } } } public class TestAnimalProcessor { public static void main(String[] args) { AnimalProcessor processor = new AnimalProcessor(); processor.processAnimal("Dog"); // Processing a Dog processor.processAnimal("Cat"); // Processing a Cat } }
この例では、`AnimalProcessor`クラスが特定の動物タイプを処理するために`if-else`文を使用しています。
新しい動物タイプを追加する場合は、このクラスを変更する必要があり、バグのリスクが高まります。
条件分岐によるコードの可読性の低下
条件分岐を多用すると、コードの可読性が低下します。
特に、大規模なシステムでは、条件分岐が複雑になり、コードを理解するのが難しくなります。
これにより、メンテナンスやデバッグが困難になり、開発効率が低下します。
class NotificationProcessor { void processNotification(String notificationType) { if (notificationType.equals("Email")) { System.out.println("Processing Email notification"); } else if (notificationType.equals("SMS")) { System.out.println("Processing SMS notification"); } else { System.out.println("Unknown notification type"); } } } public class TestNotificationProcessor { public static void main(String [] args) { NotificationProcessor processor = new NotificationProcessor(); processor.processNotification("Email"); // Processing Email notification processor.processNotification("SMS"); // Processing SMS notification } }
この例では、`NotificationProcessor`クラスが特定の通知タイプを処理するために`if-else`文を使用しています。
条件分岐が多くなると、コードの可読性が低下し、理解が難しくなります。
ポリモフィズムを利用した場合のコード例とその利点
ポリモフィズムを利用することで、コードの保守性、拡張性、再利用性が大幅に向上します。
異なる動作を持つオブジェクトを簡単に追加でき、コードの変更を最小限に抑えることができます。
以下は、ポリモフィズムを使用した例です。
interface Payment { void pay(int amount); } class CreditCardPayment implements Payment { public void pay(int amount) { System.out.println("Paid " + amount + " using Credit Card"); } } class PayPalPayment implements Payment { public void pay(int amount) { System.out.println("Paid " + amount + " using PayPal"); } } public class TestPayment { public static void main(String[] args) { Payment payment = new CreditCardPayment(); payment.pay(100); // Paid 100 using Credit Card payment = new PayPalPayment(); payment.pay(200); // Paid 200 using PayPal } }
この例では、`Payment`インターフェースを使用して、`CreditCardPayment`と`PayPalPayment`クラスが定義されています。
`TestPayment`クラスでは、`Payment`型の変数を使用して支払い方法を変更しています。
ポリモフィズムにより、異なる支払い方法を簡単に追加でき、コードの柔軟性が向上しています。
ポリモフィズムを使ったプログラムの利点
ポリモフィズムを使用することで、以下の利点が得られます:
1. 保守性の向上:新しい機能を追加する際に、既存のコードを変更する必要がないため、コードの保守が容易になります。
2. 拡張性の向上:新しい種類のオブジェクトを追加する際に、インターフェースを実装するだけで済むため、プログラムの拡張性が高まります。
3. コードの可読性の向上:複雑な条件分岐を避けることができ、コードの可読性が向上します。
4. バグの減少:条件分岐が少なくなるため、バグの発生リスクが低減します。
interface Shape { void draw(); } class Circle implements Shape { public void draw() { System.out.println("Drawing a Circle"); } } class Rectangle implements Shape { public void draw() { System.out.println("Drawing a Rectangle"); } } public class TestShape { public static void main(String[] args) { Shape shape = new Circle(); shape.draw(); // Drawing a Circle shape = new Rectangle(); shape.draw(); // Drawing a Rectangle } }
上記の例では、`Shape`インターフェースを使用して、`Circle`および`Rectangle`クラスが定義されています。
`TestShape`クラスでは、`Shape`型の変数を使用して形状を動的に切り替えています。
ポリモフィズムにより、異なる形状を簡単に追加でき、コードの柔軟性が向上しています。
ポリモフィズムを使ったコードの再利用性
ポリモフィズムを使用することで、コードの再利用性が向上します。
異なるクラスが同じインターフェースを実装することで、同じメソッドを異なるコンテキストで使用することが可能になります。
これにより、共通の機能を持つコードを再利用しやすくなります。
interface Printer { void print(String document); } class InkjetPrinter implements Printer { public void print(String document) { System.out.println("Printing with Inkjet: " + document); } } class LaserPrinter implements Printer { public void print(String document) { System.out.println("Printing with Laser: " + document); } } public class TestPrinter { public static void main(String[] args) { Printer printer = new InkjetPrinter(); printer.print("Document 1"); // Printing with Inkjet: Document 1 printer = new LaserPrinter(); printer.print("Document 2"); // Printing with Laser: Document 2 } }
この例では、`Printer`インターフェースを使用して、`InkjetPrinter`および`LaserPrinter`クラスが定義されています。
`TestPrinter`クラスでは、`Printer`型の変数を使用して、プリンタを動的に切り替えています。
ポリモフィズムにより、異なるプリンタが同じメソッドを実装し、異なる動作をすることが確認できます。
ポリモフィズムを使ったコードの保守性
ポリモフィズムを使用することで、コードの保守性が向上します。
新しい機能を追加する際に、既存のコードを変更する必要がないため、保守が容易になります。
また、ポリモフィズムを使用することで、変更に強い設計が可能となり、バグの発生リスクが低減します。
interface Vehicle { void start(); } class Car implements Vehicle { public void start() { System.out.println("Car starts"); } } class Motorcycle implements Vehicle { public void start() { System.out.println("Motorcycle starts"); } } public class TestVehicle { public static void main(String[] args) { Vehicle vehicle = new Car(); vehicle.start(); // Car starts vehicle = new Motorcycle(); vehicle.start(); // Motorcycle starts } }
この例では、`Vehicle`インターフェースを使用して、`Car`および`Motorcycle`クラスが定義されています。
`TestVehicle`クラスでは、`Vehicle`型の変数を使用して、車とオートバイを動的に切り替えています。
ポリモフィズムにより、異なる車両が同じメソッドを実装し、異なる動作をすることが確認できます。
ポリモフィズムを使ったコードの拡張性
ポリモフィズムを使用することで、コードの拡張性が向上します。
新しいクラスを追加する際に、インターフェースを実装するだけで済むため、既存のコードに影響を与えずに新しい機能を追加できます。
これにより、プログラムの拡張が容易になり、開発効率が向上します。
interface Notification { void send(String message); } class EmailNotification implements Notification { public void send(String message) { System.out.println("Sending email: " + message); } } class SMSNotification implements Notification { public void send(String message) { System.out.println("Sending SMS: " + message); } } class PushNotification implements Notification { public void send(String message) { System.out.println("Sending push notification: " + message); } } public class TestNotification { public static void main(String[] args) { Notification notification = new EmailNotification(); notification.send("Hello via Email"); // Sending email: Hello via Email notification = new SMSNotification(); notification.send("Hello via SMS"); // Sending SMS: Hello via SMS notification = new PushNotification(); notification.send("Hello via Push"); // Sending push notification: Hello via Push } }
この例では、`Notification`インターフェースを使用して、`EmailNotification`、`SMSNotification`、`PushNotification`クラスが定義されています。
`TestNotification`クラスでは、`Notification`型の変数を使用して通知方法を変更しています。
ポリモフィズムにより、異なる通知方法を簡単に追加でき、コードの拡張性が向上しています。
ポリモフィズムを使ったコードの柔軟性
ポリモフィズムを使用することで、コードの柔軟性が向上します。
異なる実装を同じインターフェースで扱うことができるため、プログラムの柔軟性が高まり、変更に対応しやすくなります。
これにより、開発者はコードを簡単に変更・拡張することができ、プログラムの寿命が延びます。
interface Storage { void save(String data); } class DatabaseStorage implements Storage { public void save(String data) { System.out .println("Saving data to database: " + data); } } class FileStorage implements Storage { public void save(String data) { System.out.println("Saving data to file: " + data); } } public class TestStorage { public static void main(String[] args) { Storage storage = new DatabaseStorage(); storage.save("User data"); // Saving data to database: User data storage = new FileStorage(); storage.save("User data"); // Saving data to file: User data } }
この例では、`Storage`インターフェースを使用して、`DatabaseStorage`および`FileStorage`クラスが定義されています。
`TestStorage`クラスでは、`Storage`型の変数を使用してストレージ方法を変更しています。
ポリモフィズムにより、異なるストレージ方法を簡単に追加・変更でき、コードの柔軟性が向上しています。
ポリモフィズムの応用とそのメリット
ポリモフィズムは、多くの実世界のアプリケーションで応用されています。
これにより、コードの再利用性、保守性、拡張性が大幅に向上します。
具体的な応用例としては、GUIフレームワーク、デザインパターン、ゲーム開発などが挙げられます。
ポリモフィズムを適切に活用することで、複雑なソフトウェアシステムを効率的に管理し、変更に柔軟に対応することが可能となります。
interface MediaPlayer { void play(String fileName); } class AudioPlayer implements MediaPlayer { public void play(String fileName) { System.out.println("Playing audio file: " + fileName); } } class VideoPlayer implements MediaPlayer { public void play(String fileName) { System.out.println("Playing video file: " + fileName); } } public class TestMediaPlayer { public static void main(String[] args) { MediaPlayer player = new AudioPlayer(); player.play("song.mp3"); // Playing audio file: song.mp3 player = new VideoPlayer(); player.play("movie.mp4"); // Playing video file: movie.mp4 } }
この例では、`MediaPlayer`インターフェースを使用して、`AudioPlayer`および`VideoPlayer`クラスが定義されています。
`TestMediaPlayer`クラスでは、`MediaPlayer`型の変数を使用して、再生するメディアを動的に変更しています。
ポリモフィズムにより、異なるメディアプレーヤーを簡単に追加でき、コードの柔軟性が向上しています。
GUIフレームワークにおけるポリモフィズムの応用
GUIフレームワークでは、ポリモフィズムを利用して、異なる種類のウィジェット(ボタン、テキストフィールドなど)を同じインターフェースで操作することができます。
これにより、コードの再利用性と柔軟性が向上し、異なるウィジェットを簡単に追加・変更することが可能です。
interface Widget { void render(); } class Button implements Widget { public void render() { System.out.println("Rendering a Button"); } } class TextField implements Widget { public void render() { System.out.println("Rendering a TextField"); } } public class TestWidget { public static void main(String[] args) { Widget widget = new Button(); widget.render(); // Rendering a Button widget = new TextField(); widget.render(); // Rendering a TextField } }
この例では、`Widget`インターフェースを使用して、`Button`および`TextField`クラスが定義されています。
`TestWidget`クラスでは、`Widget`型の変数を使用して、ウィジェットを動的に切り替えています。
ポリモフィズムにより、異なるウィジェットを簡単に追加・変更でき、コードの柔軟性が向上しています。
デザインパターンにおけるポリモフィズムの利用
デザインパターンの多くは、ポリモフィズムを利用して柔軟な設計を実現しています。
例えば、ストラテジーパターンでは、異なるアルゴリズムを同じインターフェースで実装し、動的に切り替えることができます。
これにより、アルゴリズムの追加や変更が容易になり、コードの保守性と拡張性が向上します。
interface Strategy { int execute(int a, int b); } class AdditionStrategy implements Strategy { public int execute(int a, int b) { return a + b; } } class SubtractionStrategy implements Strategy { public int execute(int a, int b) { return a - b; } } public class TestStrategy { public static void main(String[] args) { Strategy strategy = new AdditionStrategy(); System.out.println("Addition: " + strategy.execute(5, 3)); // Addition: 8 strategy = new SubtractionStrategy(); System.out.println("Subtraction: " + strategy.execute(5, 3)); // Subtraction: 2 } }
この例では、`Strategy`インターフェースを使用して、`AdditionStrategy`および`SubtractionStrategy`クラスが定義されています。
`TestStrategy`クラスでは、`Strategy`型の変数を使用して、アルゴリズムを動的に切り替えています。
ポリモフィズムにより、異なるアルゴリズムを簡単に追加・変更でき、コードの柔軟性が向上しています。
ゲーム開発におけるポリモフィズムの応用
ゲーム開発では、ポリモフィズムを利用して、異なる種類のキャラクターやオブジェクトを同じインターフェースで操作することができます。
これにより、キャラクターやオブジェクトの追加・変更が容易になり、ゲームの拡張性と保守性が向上します。
interface Character { void attack(); } class Warrior implements Character { public void attack() { System.out.println("Warrior attacks with a sword"); } } class Mage implements Character { public void attack() { System.out.println("Mage attacks with a spell"); } } public class TestCharacter { public static void main(String[] args) { Character character = new Warrior(); character.attack(); // Warrior attacks with a sword character = new Mage(); character.attack(); // Mage attacks with a spell } }
この例では、`Character`インターフェースを使用して、`Warrior`および`Mage`クラスが定義されています。
`TestCharacter`クラスでは、`Character`型の変数を使用して、キャラクターを動的に切り替えています。
ポリモフィズムにより、異なるキャラクターを簡単に追加・変更でき、コードの柔軟性が向上しています。
ポリモフィズムのメリットと実践的な応用
ポリモフィズムを利用することで、ソフトウェア開発において多くのメリットが得られます。
具体的には、コードの再利用性、保守性、拡張性が向上し、開発効率が大幅に改善されます。
これにより、変更に強い柔軟な設計が可能となり、実世界の複雑な問題に対処しやすくなります。
interface Document { void open(); } class WordDocument implements Document { public void open() { System.out.println("Opening Word document"); } } class PdfDocument implements Document { public void open() { System.out.println("Opening PDF document"); } } public class TestDocument { public static void main(String[] args) { Document doc = new WordDocument(); doc.open(); // Opening Word document doc = new PdfDocument(); doc.open(); // Opening PDF document } }
この例では、`Document`インターフェースを使用して、`WordDocument`および`PdfDocument`クラスが定義されています。
`TestDocument`クラスでは、`Document`型の変数を使用して、ドキュメントを動的に切り替えています。
ポリモフィズムにより、異なる種類のドキュメントを簡単に追加・変更でき、コードの柔軟性が向上しています。
ポリモフィズムの実世界での応用例
ポリモフィズムは、実世界のさまざまな場面で応用されています。
例えば、銀行システムでは、異なる種類のアカウント(普通預金、定期預金など)を同じインターフェースで扱うことができます。
これにより、新しいアカウントタイプを追加する際に、既存のコードに影響を与えることなく拡張することができます。
interface Account { void deposit(double amount); void withdraw(double amount); } class SavingsAccount implements Account { private double balance = 0; public void deposit(double amount) { balance += amount; System.out.println("Deposited " + amount + " to Savings Account. Balance: " + balance); } public void withdraw(double amount) { if (balance >= amount) { balance -= amount; System.out.println("Withdrew " + amount + " from Savings Account. Balance: " + balance); } else { System.out.println("Insufficient funds in Savings Account."); } } } class FixedDepositAccount implements Account { private double balance = 0; public void deposit(double amount) { balance += amount; System.out.println("Deposited " + amount + " to Fixed Deposit Account. Balance: " + balance); } public void withdraw(double amount) { System.out.println("Withdrawal not allowed from Fixed Deposit Account."); } } public class TestAccount { public static void main(String[] args) { Account account = new SavingsAccount(); account.deposit(500); account.withdraw(200); account = new FixedDepositAccount(); account.deposit(1000); account.withdraw(500); } }
この例では、`Account`インターフェースを使用して、`SavingsAccount`および`FixedDepositAccount`クラスが定義されています。
`TestAccount`クラスでは、`Account`型の変数を使用して、アカウントの種類を動的に変更しています。
ポリモフィズムにより、異なるアカウントタイプを簡単に追加・変更でき、コードの柔軟性が向上しています。
ポリモフィズムを利用したデザインパターンの実例
ポリモフィズムを利用したデザインパターンの一例として、ファクトリーパターンがあります。
ファクトリーパターンは、オブジェクトの生成を専用のクラスに委任することで、コードの再利用性と拡張性を向上させる設計パターンです。
interface Shape { void draw(); } class Circle implements Shape { public void draw() { System.out.println("Drawing a Circle"); } } class Rectangle implements Shape { public void draw() { System.out.println("Drawing a Rectangle"); } } class ShapeFactory { public static Shape getShape(String shapeType) { if (shapeType == null) { return null; } if (shapeType.equalsIgnoreCase("CIRCLE")) { return new Circle(); } else if (shapeType.equalsIgnoreCase("RECTANGLE")) { return new Rectangle(); } return null; } } public class TestFactory { public static void main(String[] args) { Shape shape1 = ShapeFactory.getShape("CIRCLE"); shape1.draw(); // Drawing a Circle Shape shape2 = ShapeFactory.getShape("RECTANGLE"); shape2.draw(); // Drawing a Rectangle } }
この例では、`Shape`インターフェースを使用して、`Circle`および`Rectangle`クラスが定義されています。
`ShapeFactory`クラスは、形状オブジェクトを生成するためのファクトリーメソッドを提供します。
`TestFactory`クラスでは、`ShapeFactory`を使用して形状オブジェクトを動的に生成し、それぞれの`draw`メソッドを呼び出しています。
ポリモフィズムにより、ファクトリーパターンを利用してオブジェクトの生成を柔軟に行うことができます。
ポリモフィズムを活用したリファクタリングの実践
ポリモフィズムを活用することで、既存のコードをリファクタリングして、より柔軟で保守しやすい設計にすることができます。
例えば、条件分岐が多く含まれるコードをインターフェースや継承を使って整理することで、コードの可読性と拡張性が向上します。
class DocumentProcessor { void processDocument(String documentType) { if (documentType.equals("Word")) { System.out.println("Processing Word document"); } else if (documentType.equals("PDF")) { System.out.println("Processing PDF document"); } else { System.out.println("Unknown document type"); } } } interface Document { void process(); } class WordDocument implements Document { public void process() { System.out.println("Processing Word document"); } } class PdfDocument implements Document { public void process() { System.out.println("Processing PDF document"); } } public class TestDocumentProcessor { public static void main(String[] args) { Document doc = new WordDocument(); doc.process(); // Processing Word document doc = new PdfDocument(); doc.process(); // Processing PDF document } }
この例では、`DocumentProcessor`クラスが条件分岐を使用してドキュメントを処理する元の実装を示しています。
その後、`Document`インターフェースを使用して、`WordDocument`および`PdfDocument`クラスが定義されています。
`TestDocumentProcessor`クラスでは、`Document`型の変数を使用してドキュメントの種類を動的に変更し、それぞれの`process`メソッドを呼び出しています。
ポリモフィズムにより、条件分岐を削減し、コードの可読性と保守性が向上しています。