ヘキサゴナルアーキテクチャとは何か?その基本概念と特徴を詳しく解説
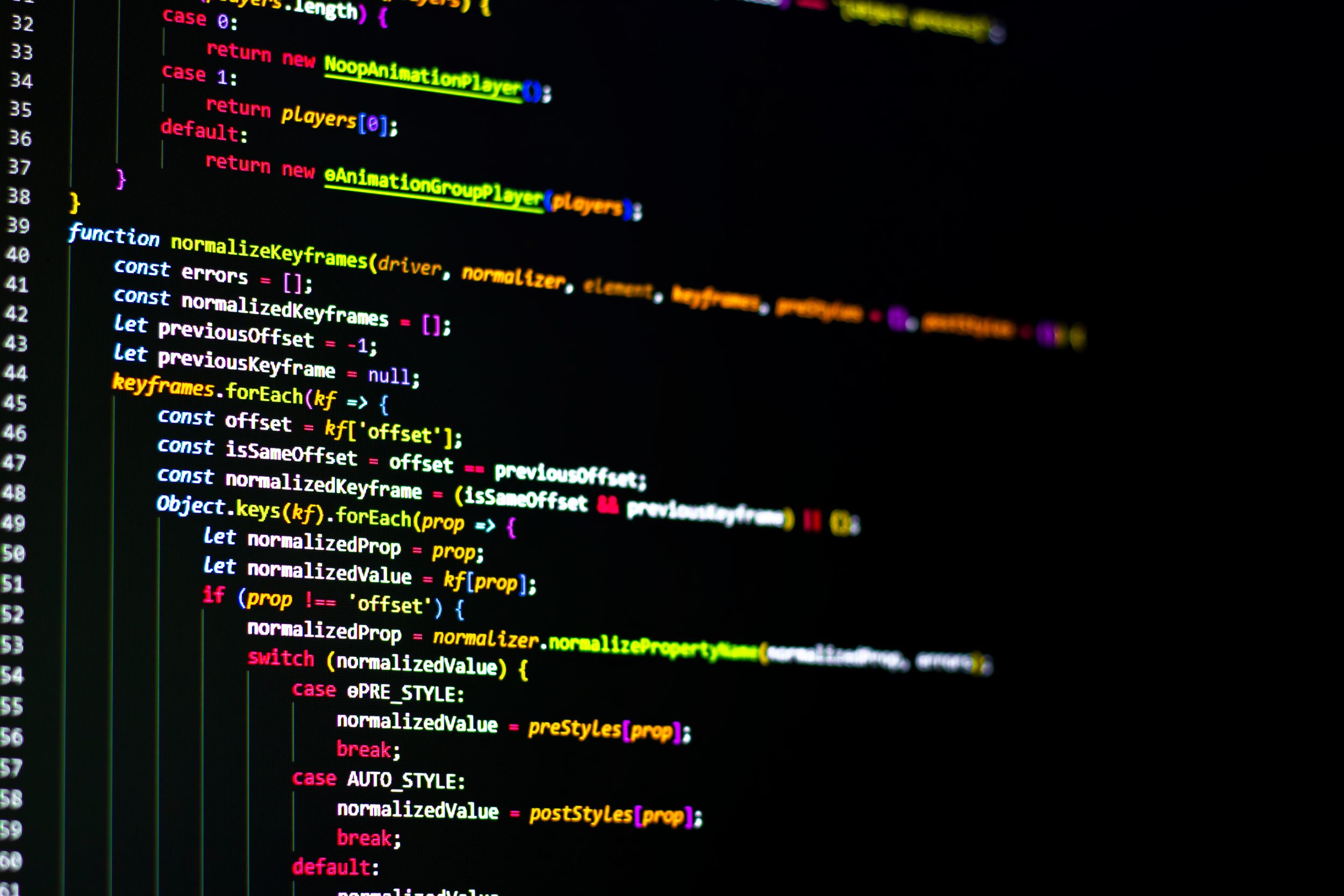
目次
ヘキサゴナルアーキテクチャとは何か?その基本概念と特徴を詳しく解説
ヘキサゴナルアーキテクチャ(Hexagonal Architecture)は、ソフトウェア設計において柔軟性とテスタビリティを高めるためのアーキテクチャスタイルです。
ポートアンドアダプターアーキテクチャ(Ports and Adapters Architecture)とも呼ばれ、システムの内部と外部を分離し、異なる技術スタックや外部システムとのインターフェースを容易に変更できることを目指しています。
基本概念として、中心にビジネスロジックを置き、その周りをポート(インターフェース)とアダプター(具体的な実装)が取り囲む構造を持ちます。
ヘキサゴナルアーキテクチャの基本概念とは?
ヘキサゴナルアーキテクチャの基本概念は、システムのコアであるビジネスロジックと、それを取り巻くインターフェース(ポート)およびその実装(アダプター)に分離することです。
これにより、ビジネスロジックはインフラストラクチャの変更に影響されず、容易にテスト可能となります。
# サンプルコード class Order: def __init__(self, id, amount): self.id = id self.amount = amount class OrderRepository: def save(self, order: Order): raise NotImplementedError class OrderService: def __init__(self, repository: OrderRepository): self.repository = repository def create_order(self, id, amount): order = Order(id, amount) self.repository.save(order) # インフラ層の具体的な実装例 class InMemoryOrderRepository(OrderRepository): def __init__(self): self.orders = [] def save(self, order: Order): self.orders.append(order) print(f"Order {order.id} saved with amount {order.amount}") # アプリケーションの実行 repository = InMemoryOrderRepository() service = OrderService(repository) service.create_order(1, 100)
ヘキサゴナルアーキテクチャの歴史と背景
ヘキサゴナルアーキテクチャは、2005年にAlistair Cockburnによって提唱されました。
従来のレイヤードアーキテクチャの問題点、特に外部システムやインフラストラクチャの変更に弱いという課題を解決するために考案されました。
このアーキテクチャは、システムの柔軟性と保守性を向上させるために、ビジネスロジックを中心に置き、周辺の技術的な詳細を切り離すことを目的としています。
ヘキサゴナルアーキテクチャの主要な構成要素
ヘキサゴナルアーキテクチャの主要な構成要素は以下の通りです:
– ドメイン(ビジネスロジック):システムの核心部分で、ビジネスルールやエンティティを含みます。
– ポート(インターフェース):ドメインと外部システムを繋ぐインターフェースで、入出力の抽象化を行います。
– アダプター(実装):ポートを通じて外部システムと連携する具体的な実装部分です。
データベースアクセス、外部APIの呼び出し、ユーザーインターフェースなどが含まれます。
ヘキサゴナルアーキテクチャの利点と欠点
ヘキサゴナルアーキテクチャの利点は以下の通りです:
– 柔軟性:技術スタックの変更や新しいインターフェースの追加が容易です。
– テスタビリティ:ビジネスロジックを独立してテストできるため、テストがしやすくなります。
– 保守性:ビジネスロジックと技術的な詳細が分離されているため、コードの理解と修正がしやすいです。
一方、欠点も存在します:
– 複雑性:構成要素が多いため、設計と実装に時間と労力がかかります。
– オーバーヘッド:小規模なプロジェクトには過剰なアプローチとなる場合があります。
ヘキサゴナルアーキテクチャの実装例
以下に、ヘキサゴナルアーキテクチャを用いたシンプルな実装例を示します。
# ドメイン層 class Product: def __init__(self, id, name, price): self.id = id self.name = name self.price = price class ProductRepository: def add(self, product: Product): raise NotImplementedError # アプリケーション層 class ProductService: def __init__(self, repository: ProductRepository): self.repository = repository def add_product(self, id, name, price): product = Product(id, name, price) self.repository.add(product) # インフラ層 class InMemoryProductRepository(ProductRepository): def __init__(self): self.products = [] def add(self, product: Product): self.products.append(product) print(f"Product {product.name} added with price {product.price}") # アダプタ層 repository = InMemoryProductRepository() service = ProductService(repository) service.add_product(1, "Laptop", 1500)
このコードは、ドメイン層、アプリケーション層、インフラ層の分離を示しており、各層の役割と実装方法を理解するのに役立ちます。
ヘキサゴナルアーキテクチャとクリーンアーキテクチャの違いを理解する
ヘキサゴナルアーキテクチャとクリーンアーキテクチャは、どちらも柔軟性とテスタビリティを高めることを目的としたアーキテクチャスタイルですが、アプローチと構造に違いがあります。
クリーンアーキテクチャは、Robert C. Martinによって提唱され、レイヤードアーキテクチャの進化形として位置づけられます。
クリーンアーキテクチャの基本概念と特徴
クリーンアーキテクチャの基本概念は、システムを複数のレイヤーに分割し、各レイヤーが特定の責任を持つことです。
特に、ビジネスロジック(ユースケース)を中心に据え、外部の詳細(UI、データベース、外部システム)から独立させることを重視します。
# サンプルコード class UseCase: def execute(self, request): raise NotImplementedError class AddProductUseCase(UseCase): def __init__(self, repository): self.repository = repository def execute(self, request): product = Product(request.id, request.name, request.price) self.repository.add(product) class ProductRepository: def add(self, product): raise NotImplementedError # プレゼンテーション層 class AddProductController: def __init__(self, use_case): self.use_case = use_case def handle(self, request): self.use_case.execute(request) # インフラ層 class InMemoryProductRepository(ProductRepository): def __init__(self): self.products = [] def add(self, product): self.products.append(product) print(f"Product {product.name} added with price {product.price}") # 実行例 repository = InMemoryProductRepository() use_case = AddProductUseCase(repository) controller = AddProductController(use_case) request = type("Request", (object,), {"id": 1, "name": "Laptop", "price": 1500}) controller.handle(request)
ヘキサゴナルアーキテクチャとクリーンアーキテクチャの共通点
両アーキテクチャは以下の共通点を持っています:
– テスタビリティの向上:ビジネスロジックを独立してテストできるように設計されています。
– 柔軟性の確保:
システムの各部分が疎結合となるように設計されています。
– 保守性の向上:コードの理解と修正が容易になるように構成されています。
ヘキサゴナルアーキテクチャとクリーンアーキテクチャの相違点
相違点としては、以下が挙げられます:
– 構造の違い:ヘキサゴナルアーキテクチャはポートとアダプターの概念に基づいていますが、クリーンアーキテクチャはレイヤーによる分割を重視します。
– 中心の違い:ヘキサゴナルアーキテクチャはビジネスロジックを中心に据えますが、クリーンアーキテクチャはユースケースを中心に据えます。
どちらのアーキテクチャを選ぶべきか?
プロジェクトの規模や要件によって、どちらのアーキテクチャを選ぶかが決まります。
柔軟性やテスタビリティを重視する場合は、どちらも優れた選択肢ですが、特定の要件や技術スタックに応じて最適なものを選ぶことが重要です。
クリーンアーキテクチャの実装例
以下に、クリーンアーキテクチャを用いたシンプルな実装例を示します。
# ドメイン層 class Product: def __init__(self, id, name, price): self.id = id self.name = name self.price = price class ProductRepository: def add(self, product): raise NotImplementedError # アプリケーション層 class AddProductUseCase: def __init__(self, repository): self.repository = repository def execute(self, request): product = Product(request.id, request.name, request.price) self.repository.add(product) # プレゼンテーション層 class AddProductController: def __init__(self, use_case): self.use_case = use_case def handle(self, request): self.use_case.execute(request) # インフラ層 class InMemoryProductRepository(ProductRepository): def __init__(self): self.products = [] def add(self, product): self.products.append(product) print(f"Product {product.name} added with price {product.price}") # 実行例 repository = InMemoryProductRepository() use_case = AddProductUseCase(repository) controller = AddProductController(use_case) request = type("Request", (object,), {"id": 1, "name": "Laptop", "price": 1500}) controller.handle(request)
このコードは、クリーンアーキテクチャのレイヤー構造を示しており、各レイヤーの役割と実装方法を理解するのに役立ちます。
ヘキサゴナルアーキテクチャとオニオンアーキテクチャの比較と使い分け
ヘキサゴナルアーキテクチャとオニオンアーキテクチャは、いずれもソフトウェアの柔軟性とテスタビリティを向上させることを目指したアーキテクチャスタイルです。
しかし、それぞれのアプローチや構造には違いがあります。
このセクションでは、両者の特徴を比較し、どのように使い分けるべきかを解説します。
オニオンアーキテクチャの基本概念と特徴
オニオンアーキテクチャ(Onion Architecture)は、Jeffrey Palermoによって提唱されたアーキテクチャスタイルです。
ビジネスロジックを中心に据え、外部からの依存を最小限に抑えることを目的としています。
オニオンアーキテクチャの特徴は、中心から外側へ向かって、ドメイン層、アプリケーション層、インフラストラクチャ層の順に構成されることです。
# ドメイン層 class User: def __init__(self, id, name): self.id = id self.name = name class UserRepository: def add(self, user: User): raise NotImplementedError # アプリケーション層 class UserService: def __init__(self, repository: UserRepository): self.repository = repository def add_user(self, id, name): user = User(id, name) self.repository.add(user) # インフラストラクチャ層 class InMemoryUserRepository(UserRepository): def __init__(self): self.users = [] def add(self, user: User): self.users.append(user) print(f"User {user.name} added with ID {user.id}") # アプリケーションの実行 repository = InMemoryUserRepository() service = UserService(repository) service.add_user(1, "Alice")
ヘキサゴナルアーキテクチャとオニオンアーキテクチャの共通点
両アーキテクチャは、ソフトウェアの保守性とテスタビリティを向上させることを目的としています。
以下の点で共通しています:
– 中心にビジネスロジックを据える:どちらのアーキテクチャも、ビジネスロジックを中心に置き、外部の詳細から独立させています。
– 柔軟な設計:技術スタックや外部システムの変更に対して柔軟であり、システムの拡張が容易です。
– テストのしやすさ:ビジネスロジックが独立しているため、単体テストが容易です。
ヘキサゴナルアーキテクチャとオニオンアーキテクチャの相違点
相違点は以下の通りです:
– 構造の違い:ヘキサゴナルアーキテクチャはポートとアダプターを中心に据えていますが、オニオンアーキテクチャはドメイン層を中心にレイヤーを構成しています。
– 依存関係の扱い:ヘキサゴナルアーキテクチャは、ポートを通じて外部システムとの依存関係を管理しますが、オニオンアーキテクチャはドメイン層に依存する形で外側にレイヤーを重ねます。
プロジェクトに応じたアーキテクチャの選択方法
プロジェクトの要件や規模によって、適切なアーキテクチャを選択することが重要です。
ヘキサゴナルアーキテクチャは、外部システムとの連携が多いプロジェクトに向いています。
一方、オニオンアーキテクチャは、ビジネスロジックが複雑で、ドメイン層を中心に据えた設計が必要なプロジェクトに適しています。
オニオンアーキテクチャの実装例
以下に、オニオンアーキテクチャを用いたシンプルな実装例を示します。
# ドメイン層 class Task: def __init__(self, id, description): self.id = id self.description = description class TaskRepository: def save(self, task: Task): raise NotImplementedError # アプリケーション層 class TaskService: def __init__(self, repository: TaskRepository): self.repository = repository def create_task(self, id, description): task = Task(id, description) self.repository.save(task) # インフラストラクチャ層 class InMemoryTaskRepository(TaskRepository): def __init__(self): self.tasks = [] def save(self, task: Task): self.tasks.append(task) print(f"Task {task.description} added with ID {task.id}") # アプリケーションの実行 repository = InMemoryTaskRepository() service = TaskService(repository) service.create_task(1, "Implement Onion Architecture")
このコードは、オニオンアーキテクチャのレイヤー構造を示しており、各レイヤーの役割と実装方法を理解するのに役立ちます。
実際のプロジェクトにおけるヘキサゴナルアーキテクチャのサンプルコード
ヘキサゴナルアーキテクチャを実際のプロジェクトに適用する際には、各層の役割を明確にし、ポートとアダプターの設計を行うことが重要です。
ここでは、簡単なサンプルプロジェクトを通じて、ヘキサゴナルアーキテクチャの具体的な実装方法を解説します。
サンプルプロジェクトの概要と設計方針
今回のサンプルプロジェクトでは、シンプルなタスク管理システムを構築します。
システムは以下のコンポーネントから構成されます:
– ドメイン層:タスクエンティティとそのビジネスロジックを含む。
– アプリケーション層:タスクの作成、取得、削除などのユースケースを実装。
– インフラストラクチャ層:データベースアクセスや外部APIとの連携を実装。
– アダプタ層:ユーザーインターフェースや外部システムとのインターフェースを提供。
# ドメイン層 class Task: def __init__(self, id, title, description): self.id = id self.title = title self.description = description class TaskRepository: def save(self, task: Task): raise NotImplementedError # アプリケーション層 class TaskService: def __init__(self, repository: TaskRepository): self.repository = repository def create_task(self, id, title, description): task = Task(id, title, description) self.repository.save(task) # インフラストラクチャ層 class InMemoryTaskRepository(TaskRepository): def __init__(self): self.tasks = [] def save(self, task: Task): self.tasks.append(task) print(f"Task '{task.title}' added with description: {task.description}") # アダプタ層 class TaskController: def __init__(self, service: TaskService): self.service = service def create_task(self, id, title, description): self.service.create_task(id, title, description) # アプリケーションの実行 repository = InMemoryTaskRepository() service = TaskService(repository) controller = TaskController(service) controller.create_task(1, "Learn Hexagonal Architecture", "Understand the basics of Hexagonal Architecture.")
ドメイン層の実装例
ドメイン層では、ビジネスロジックとエンティティを定義します。
タスク管理システムの例では、タスクエンティティとその振る舞いを定義します。
class Task: def __init__(self, id, title, description): self.id = id self.title = title self.description = description def update_description(self, new_description): self.description = new_description
アプリケーション層の実装例
アプリケーション層では、ユース
ケースを実装します。
タスクの作成や更新などのビジネスロジックを実装します。
class TaskService: def __init__(self, repository: TaskRepository): self.repository = repository def create_task(self, id, title, description): task = Task(id, title, description) self.repository.save(task) def update_task_description(self, id, new_description): task = self.repository.find_by_id(id) if task: task.update_description(new_description) self.repository.save(task)
インフラストラクチャ層の実装例
インフラストラクチャ層では、データベースアクセスや外部システムとの連携を実装します。
ここでは、インメモリのリポジトリを例として示します。
class InMemoryTaskRepository(TaskRepository): def __init__(self): self.tasks = [] def save(self, task: Task): self.tasks.append(task) print(f"Task '{task.title}' saved with description: {task.description}") def find_by_id(self, id): return next((task for task in self.tasks if task.id == id), None)
アダプタ層の実装例
アダプタ層では、ユーザーインターフェースや外部システムとのインターフェースを提供します。
ここでは、簡単なコントローラを示します。
class TaskController: def __init__(self, service: TaskService): self.service = service def create_task(self, id, title, description): self.service.create_task(id, title, description) def update_task_description(self, id, new_description): self.service.update_task_description(id, new_description) # アプリケーションの実行例 repository = InMemoryTaskRepository() service = TaskService(repository) controller = TaskController(service) controller.create_task(1, "Learn Hexagonal Architecture", "Understand the basics of Hexagonal Architecture.") controller.update_task_description(1, "Master Hexagonal Architecture.")
これらのコード例を通じて、ヘキサゴナルアーキテクチャの各層の役割と実装方法を具体的に理解できます。
ヘキサゴナルアーキテクチャを用いたDDDの実践方法
ヘキサゴナルアーキテクチャ(Hexagonal Architecture)は、ドメイン駆動設計(DDD)との親和性が高く、ビジネスロジックを中心に据えたシステム設計を実現するための強力なツールです。
このセクションでは、ヘキサゴナルアーキテクチャを用いたDDDの実践方法を解説します。
ドメイン駆動設計(DDD)の基本概念とメリット
ドメイン駆動設計(Domain-Driven Design, DDD)は、ビジネスの複雑な要件を反映したソフトウェアシステムを設計するためのアプローチです。
DDDの基本概念には、エンティティ、値オブジェクト、アグリゲート、リポジトリ、サービス、ドメインイベントなどが含まれます。
これにより、ビジネスロジックを明確にし、システム全体の整合性と一貫性を保つことができます。
# ドメイン層の実装例 class Product: def __init__(self, id, name, price): self.id = id self.name = name self.price = price class ProductRepository: def save(self, product: Product): raise NotImplementedError # アプリケーション層のサービス class ProductService: def __init__(self, repository: ProductRepository): self.repository = repository def add_product(self, id, name, price): product = Product(id, name, price) self.repository.save(product)
ヘキサゴナルアーキテクチャとDDDの親和性
ヘキサゴナルアーキテクチャは、DDDの基本概念を実装するための理想的なフレームワークです。
ポートとアダプターの構造は、ドメインロジックを中心に据えた設計を容易にし、外部システムとのインターフェースを柔軟に変更できます。
これにより、ビジネスロジックがインフラストラクチャの詳細に依存しなくなり、システムの保守性と拡張性が向上します。
DDDのコンテキストマッピングとヘキサゴナルアーキテクチャ
DDDの重要な概念の一つにコンテキストマッピングがあります。
コンテキストマッピングは、複雑なシステムにおける異なるドメインモデルやバウンデッドコンテキストの関係を明確にする方法です。
ヘキサゴナルアーキテクチャでは、各バウンデッドコンテキストを独立したモジュールとして実装し、ポートとアダプターを通じて相互作用させることができます。
# サンプルコード class Order: def __init__(self, id, total): self.id = id self.total = total class OrderRepository: def save(self, order: Order): raise NotImplementedError class PaymentService: def process_payment(self, order_id, amount): raise NotImplementedError # アプリケーション層のサービス class OrderService: def __init__(self, order_repository: OrderRepository, payment_service: PaymentService): self.order_repository = order_repository self.payment_service = payment_service def place_order(self, order: Order): self.order_repository.save(order) self.payment_service.process_payment(order.id, order.total)
DDDのユビキタス言語とヘキサゴナルアーキテクチャの統合
ユビキタス言語(Ubiquitous Language)は、DDDの中心的な概念であり、開発者とビジネス専門家が共通の言語を用いてコミュニケーションを行うことを指します。
ヘキサゴナルアーキテクチャでは、このユビキタス言語をコードに反映することで、ビジネスロジックが明確で理解しやすいものとなります。
DDDの実装例におけるヘキサゴナルアーキテクチャの適用
以下に、DDDの概念をヘキサゴナルアーキテクチャに統合した実装例を示します。
この例では、注文と支払いのプロセスを管理するシステムを実装しています。
# ドメイン層 class Customer: def __init__(self, id, name, email): self.id = id self.name = name self.email = email class CustomerRepository: def save(self, customer: Customer): raise NotImplementedError # アプリケーション層 class CustomerService: def __init__(self, repository: CustomerRepository): self.repository = repository def register_customer(self, id, name, email): customer = Customer(id, name, email) self.repository.save(customer) # インフラストラクチャ層 class InMemoryCustomerRepository(CustomerRepository): def __init__(self): self.customers = [] def save(self, customer: Customer): self.customers.append(customer) print(f"Customer {customer.name} added with email {customer.email}") # アダプタ層 class CustomerController: def __init__(self, service: CustomerService): self.service = service def register_customer(self, id, name, email): self.service.register_customer(id, name, email) # アプリケーションの実行例 repository = InMemoryCustomerRepository() service = CustomerService(repository) controller = CustomerController(service) controller.register_customer(1, "Bob", "bob@example.com")
このコードは、ヘキサゴナルアーキテクチャを用いたDDDの具体的な実装方法を示しており、各層の役割と統合方法を理解するのに役立ちます。
Golangでのヘキサゴナルアーキテクチャの実装手法とベストプラクティス
Golangは、そのシンプルさとパフォーマンスの高さから、多くの開発者に愛用されるプログラミング言語です。
ここでは、Golangを用いてヘキサゴナルアーキテクチャを実装する方法と、ベストプラクティスを紹介します。
Golangの特性とヘキサゴナルアーキテクチャの適用方法
Golangは、静的型付け、ガベージコレクション、ネイティブコンパイルを特徴とするプログラミング言語です。
そのシンプルな構文と高速な実行性能は、ヘキサゴナルアーキテクチャの実装に適しています。
以下のコード例は、Golangでの基本的なヘキサゴナルアーキテクチャの実装を示します。
// ドメイン層 type Task struct { ID int Title string Description string } // リポジトリインターフェース type TaskRepository interface { Save(task Task) error } // アプリケーション層 type TaskService struct { repository TaskRepository } func (s *TaskService) CreateTask(id int, title, description string) error { task := Task{ID: id, Title: title, Description: description} return s.repository.Save(task) } // インフラ層 type InMemoryTaskRepository struct { tasks []Task } func (r *InMemoryTaskRepository) Save(task Task) error { r.tasks = append(r.tasks, task) fmt.Printf("Task '%s' added with description: %s\n", task.Title, task.Description) return nil } // アプリケーションの実行例 func main() { repository := &InMemoryTaskRepository{} service := &TaskService{repository: repository} service.CreateTask(1, "Learn Go", "Study the basics of Golang") }
Golangによるドメイン層の設計と実装
Golangのドメイン層では、ビジネスロジックを表現する構造体とインターフェースを定義します。
以下の例では、タスクエンティティとその操作を定義しています。
package domain type Task struct { ID int Title string Description string } func (t *Task) UpdateDescription(newDescription string) { t.Description = newDescription }
Golangによるアプリケーション層の設計と実装
アプリケーション層では、ドメ
インロジックを操作するサービスを実装します。
以下の例では、タスクの作成と更新を行うサービスを示します。
package application import "example.com/domain" type TaskService struct { repository domain.TaskRepository } func (s *TaskService) CreateTask(id int, title, description string) error { task := domain.Task{ID: id, Title: title, Description: description} return s.repository.Save(task) } func (s *TaskService) UpdateTaskDescription(id int, newDescription string) error { task, err := s.repository.FindByID(id) if err != nil { return err } task.UpdateDescription(newDescription) return s.repository.Save(task) }
Golangによるインフラストラクチャ層の設計と実装
インフラストラクチャ層では、データベースアクセスや外部システムとの連携を実装します。
以下の例では、インメモリのリポジトリを示します。
package infrastructure import "example.com/domain" type InMemoryTaskRepository struct { tasks []domain.Task } func (r *InMemoryTaskRepository) Save(task domain.Task) error { r.tasks = append(r.tasks, task) fmt.Printf("Task '%s' saved with description: %s\n", task.Title, task.Description) return nil } func (r *InMemoryTaskRepository) FindByID(id int) (domain.Task, error) { for _, task := range r.tasks { if task.ID == id { return task, nil } } return domain.Task{}, fmt.Errorf("Task not found") }
Golangによるアダプタ層の設計と実装
アダプタ層では、ユーザーインターフェースや外部システムとのインターフェースを提供します。
以下の例では、簡単なコントローラを示します。
package adapter import "example.com/application" type TaskController struct { service application.TaskService } func (c *TaskController) CreateTask(id int, title, description string) error { return c.service.CreateTask(id, title, description) } func (c *TaskController) UpdateTaskDescription(id int, newDescription string) error { return c.service.UpdateTaskDescription(id, newDescription) } // アプリケーションの実行例 func main() { repository := &infrastructure.InMemoryTaskRepository{} service := &application.TaskService{Repository: repository} controller := &adapter.TaskController{Service: service} controller.CreateTask(1, "Learn Hexagonal Architecture in Go", "Study how to implement Hexagonal Architecture in Golang") controller.UpdateTaskDescription(1, "Master Hexagonal Architecture in Go") }
このコード例を通じて、Golangを用いたヘキサゴナルアーキテクチャの各層の役割と実装方法を具体的に理解できます。
Pythonでのヘキサゴナルアーキテクチャの導入とその利点
Pythonは、そのシンプルさと豊富なライブラリによって、多くの開発者に支持されています。
ここでは、Pythonでヘキサゴナルアーキテクチャを導入する方法と、その利点について解説します。
Pythonの特性とヘキサゴナルアーキテクチャの適用方法
Pythonは、動的型付け、簡潔な構文、強力な標準ライブラリを特徴とするプログラミング言語です。
これらの特性は、ヘキサゴナルアーキテクチャの導入に非常に適しています。
以下のコード例は、Pythonでの基本的なヘキサゴナルアーキテクチャの実装を示します。
# ドメイン層 class User: def __init__(self, user_id, name, email): self.user_id = user_id self.name = name self.email = email class UserRepository: def save(self, user: User): raise NotImplementedError # アプリケーション層 class UserService: def __init__(self, repository: UserRepository): self.repository = repository def register_user(self, user_id, name, email): user = User(user_id, name, email) self.repository.save(user) # インフラストラクチャ層 class InMemoryUserRepository(UserRepository): def __init__(self): self.users = [] def save(self, user: User): self.users.append(user) print(f"User {user.name} added with email {user.email}") # アダプタ層 class UserController: def __init__(self, service: UserService): self.service = service def register_user(self, user_id, name, email): self.service.register_user(user_id, name, email) # アプリケーションの実行 repository = InMemoryUserRepository() service = UserService(repository) controller = UserController(service) controller.register_user(1, "Alice", "alice@example.com")
Pythonによるドメイン層の設計と実装
Pythonのドメイン層では、ビジネスロジックを表現するクラスとインターフェースを定義します。
以下の例では、ユーザークラスとその操作を定義しています。
class User: def __init__(self, user_id, name, email): self.user_id = user_id self.name = name self.email = email def update_email(self, new_email): self.email = new_email
Pythonによるアプリケーション層の設計と実装
アプリケーション層では、ドメインロジックを操作するサービスを実装します。
以下の例では、ユーザーの登録やメールアドレスの更新を行うサービスを示します。
class UserService: def __init__(self, repository: UserRepository): self.repository = repository def register_user(self, user_id, name, email): user = User(user_id, name, email) self.repository.save(user) def update_user_email(self, user_id, new_email): user = self.repository.find_by_id(user_id) if user: user.update_email(new_email) self.repository.save(user)
Pythonによるインフラストラクチャ層の設計と実装
インフラストラクチャ層では、データベースアクセスや外部システムとの連携を実装します。
以下の例では、インメモリのリポジトリを示します。
class InMemoryUserRepository(UserRepository): def __init__(self): self.users = [] def save(self, user: User): self.users.append(user) print(f"User {user.name} saved with email {user.email}") def find_by_id(self, user_id): return next((user for user in self.users if user.user_id == user_id), None)
Pythonによるアダプタ層の設計と実装
アダプタ層では、ユーザーインターフェースや外部システムとのインターフェースを提供します。
以下の例では、簡単なコントローラを示します。
class UserController: def __init__(self, service: UserService): self.service = service def register_user(self, user_id, name, email): self.service.register_user(user_id, name, email) def update_user_email(self, user_id, new_email): self.service.update_user_email(user_id, new_email) # アプリケーションの実行例 repository = InMemoryUserRepository() service = UserService(repository) controller = UserController(service) controller.register_user(1, "Bob", "bob@example.com") controller.update_user_email(1, "newbob@example.com")
このコード例を通じて、Pythonを用いたヘキサゴナルアーキテクチャの各層の役割と実装方法を具体的に理解できます。
TypeScriptで実現するヘキサゴナルアーキテクチャのアプローチ
TypeScriptは、JavaScriptのスーパーセットとして静的型付けを提供し、大規模なプロジェクトでの開発を容易にします。
ここでは、TypeScriptを用いたヘキサゴナルアーキテクチャの導入方法とその利点について解説します。
TypeScriptの特性とヘキサゴナルアーキテクチャの適用方法
TypeScriptは、JavaScriptの柔軟さを維持しつつ、型安全性を提供します。
これにより、ヘキサゴナルアーキテクチャの導入が容易になり、コードの保守性と可読性が向上します。
以下のコード例は、TypeScriptでの基本的なヘキサゴナルアーキテクチャの実装を示します。
// ドメイン層 class Product { constructor(public id: number, public name: string, public price: number) {} } interface ProductRepository { save(product: Product): void; } // アプリケーション層 class ProductService { constructor(private repository: ProductRepository) {} addProduct(id: number, name: string, price: number): void { const product = new Product(id, name, price); this.repository.save(product); } } // インフラストラクチャ層 class InMemoryProductRepository implements ProductRepository { private products: Product[] = []; save(product: Product): void { this.products.push(product); console.log(`Product ${product.name} added with price ${product.price}`); } } // アダプタ層 class ProductController { constructor(private service: ProductService) {} createProduct(id: number, name: string, price: number): void { this.service.addProduct(id, name, price); } } // アプリケーションの実行 const repository = new InMemoryProductRepository(); const service = new ProductService(repository); const controller = new ProductController(service); controller.createProduct(1, "Laptop", 1500);
TypeScriptによるドメイン層の設計と実装
TypeScriptのドメイン層では、ビジネスロジックを表現するクラスとインターフェースを定義します。
以下の例では、プロダクトクラスとその操作を定義しています。
class Product { constructor(public id: number, public name: string, public price: number) {} updatePrice(newPrice: number): void { this.price = newPrice; } }
TypeScriptによるアプリケーション層の設計と実装
アプリケーション層では、ドメインロジックを操作するサービスを実装します。
以下の例では、プロダクトの追加や価格の更新を行うサービスを示します。
class ProductService { constructor(private repository: ProductRepository) {} addProduct(id: number, name: string, price: number): void { const product = new Product(id, name, price); this.repository.save(product); } updateProductPrice(id: number, newPrice: number): void { const product = this.repository.findById(id); if (product) { product.updatePrice(newPrice); this.repository.save(product); } } }
TypeScriptによるインフラストラクチャ層の設計と実装
インフラストラクチャ層では、データベースアクセスや外部システムとの連携を実装します。
以下の例では、インメモリのリポジトリを示します。
class InMemoryProductRepository implements ProductRepository { private products: Product[] = []; save(product: Product): void { this.products.push(product); console.log(`Product ${product.name} saved with price ${product.price}`); } findById(id: number): Product | undefined { return this.products.find(product => product.id === id); } }
TypeScriptによるアダプタ層の設計と実装
アダプタ層では、ユーザーインターフェースや外部システムとのインターフェースを提供します。
以下の例では、簡単なコントローラを示します。
class ProductController { constructor(private service: ProductService) {} createProduct(id: number, name: string, price: number): void { this.service.addProduct(id, name, price); } updateProductPrice(id: number, newPrice: number): void { this.service.updateProductPrice(id, newPrice); } } // アプリケーションの実行例 const repository = new InMemoryProductRepository(); const service = new ProductService(repository); const controller = new ProductController(service); controller.createProduct(1, "Smartphone", 800); controller.updateProductPrice(1, 850);
このコード例を通じて、TypeScriptを用いたヘキサゴナルアーキテクチャの各層の役割と実装方法を具体的に理解できます。