Dockerを用いたFastAPIとReactのコンテナ化とデプロイ方法
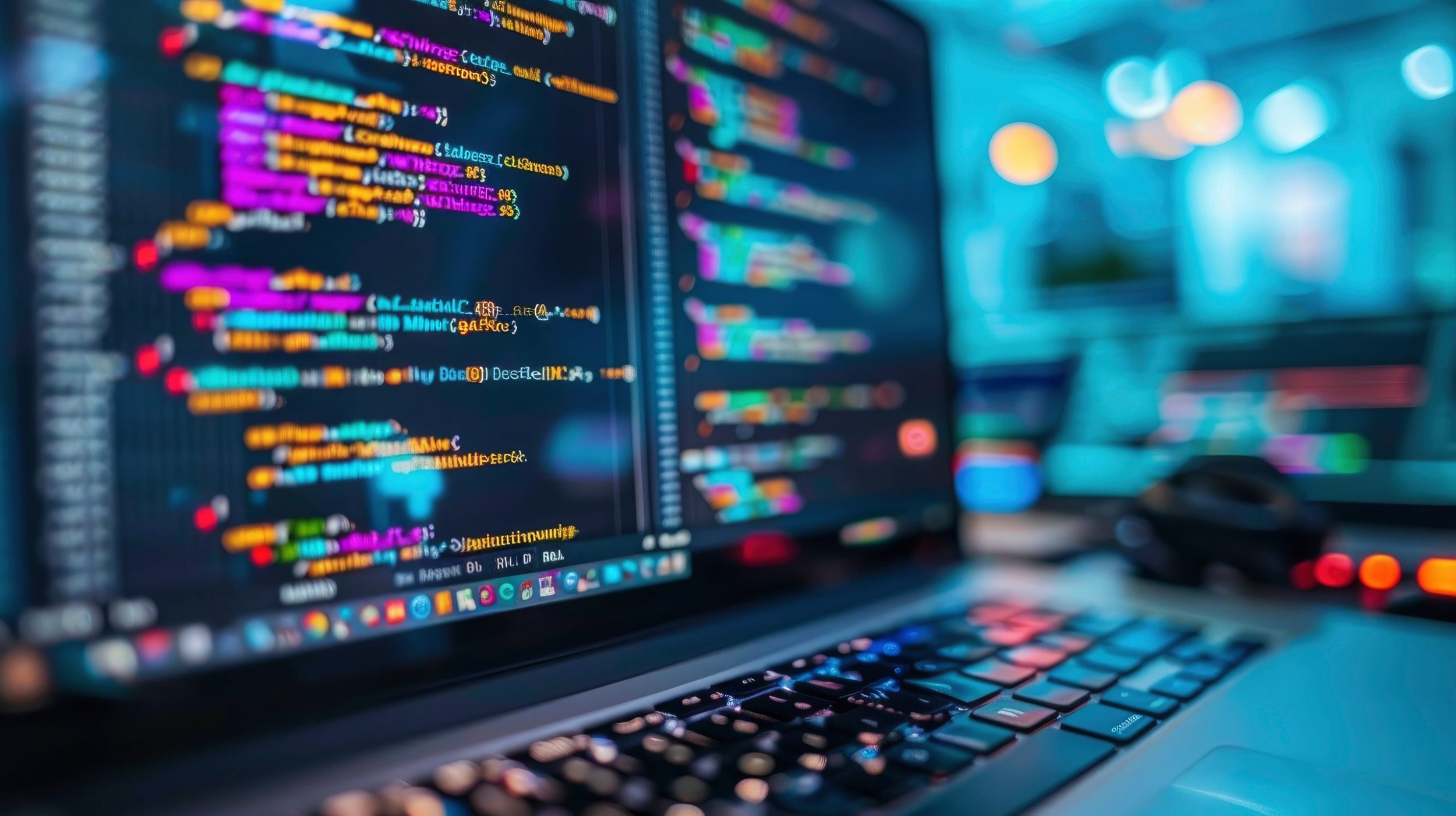
目次
ReactとFastAPIの連携方法:フロントエンドとバックエンドの統合ガイド
ReactとFastAPIの連携は、モダンなWebアプリケーション開発において非常に効果的です。
Reactはフロントエンドのユーザーインターフェースを構築するための強力なライブラリであり、一方、FastAPIは高速なバックエンドAPIを提供するためのPythonフレームワークです。
この記事では、ReactとFastAPIを連携させて効率的なWebアプリケーションを構築する方法について詳しく解説します。
まず、FastAPIでバックエンドAPIを構築し、そのAPIをReactから呼び出す基本的な方法を紹介します。
また、連携のメリットとデメリットについても触れ、最適なアプローチを選択するためのガイドラインを提供します。
以下は、FastAPIとReactを連携させるためのサンプルコードです。
FastAPIのサンプルコード
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str description: str = None @app.get("/") def read_root(): return {"Hello": "World"} @app.post("/items/") def create_item(item: Item): return {"name": item.name, "description": item.description}
Reactのサンプルコード
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [items, setItems] = useState([]); useEffect(() => { axios.get('http://localhost:8000/') .then(response => { setItems(response.data); }) .catch(error => { console.error("There was an error fetching the data!", error); }); }, []); return ( <div> <h1>Items</h1> {items.map((item, index) => ( <div key={index}> <h2>{item.name}</h2> <p>{item.description}</p> </div> ))} </div> ); } export default App;
ReactとFastAPIの基本的な概要
Reactは、ユーザーインターフェースを構築するためのJavaScriptライブラリであり、Facebookによって開発されました。
コンポーネントベースのアーキテクチャを採用しており、再利用可能なUIコンポーネントを作成するのに非常に適しています。
Reactを使用することで、効率的でスケーラブルなフロントエンドアプリケーションを構築することが可能です。
一方、FastAPIは、Pythonで書かれたモダンなWebフレームワークで、高速なパフォーマンスと簡便なAPI設計を特徴としています。
Swagger UIの自動生成や、非同期サポート、タイプヒントの利用など、開発者の生産性を大幅に向上させる機能を備えています。
これにより、迅速かつ安全なバックエンドAPIの構築が可能となります。
両者を組み合わせることで、フロントエンドとバックエンドの両方で最新の技術スタックを活用し、ユーザー体験の向上と開発効率の最適化を図ることができます。
FastAPIとReactの連携のメリットとデメリット
FastAPIとReactを連携させることには、以下のようなメリットがあります。
1. 高パフォーマンス:FastAPIの非同期処理とReactの仮想DOMにより、アプリケーション全体のパフォーマンスが向上します。
2. 迅速な開発:FastAPIの簡潔なコード記述とReactのコンポーネントベースアーキテクチャにより、迅速に開発を進めることができます。
3. 自動ドキュメント生成:FastAPIはSwagger UIやReDocによるAPIドキュメントの自動生成機能を持ち、APIの理解と利用が容易になります。
一方で、デメリットも存在します。
1. 学習曲線:両方の技術を習得する必要があるため、初期の学習コストがかかります。
2. 依存関係管理:ReactとFastAPIの連携には、依存関係の管理が必要となり、複雑になる場合があります。
FastAPIでバックエンドAPIを構築する手順
FastAPIでバックエンドAPIを構築するための基本的な手順を以下に示します。
1. 環境設定:まず、Pythonの仮想環境を作成し、FastAPIと依存関係をインストールします。
python -m venv venv source venv/bin/activate pip install fastapi uvicorn
2. APIエンドポイントの定義:FastAPIを使って、必要なAPIエンドポイントを定義します。
from fastapi import FastAPI app = FastAPI() @app.get("/") def read_root(): return {"message": "Hello World"} @app.get("/items/{item_id}") def read_item(item_id: int): return {"item_id": item_id}
3. アプリケーションの起動:Uvicornを使って、FastAPIアプリケーションを起動します。
uvicorn main:app --reload
4. テスト:ブラウザまたはAPIクライアント(例:Postman)を使って、APIエンドポイントをテストします。
これにより、基本的なバックエンドAPIが構築されました。
次に、ReactからこのAPIを呼び出す方法について説明します。
Reactでフロントエンドを構築する手順
Reactを使ってフロントエンドを構築するための基本的な手順を以下に示します。
1. 環境設定:まず、Create React Appを使ってReactプロジェクトを作成します。
npx create-react-app my-app cd my-app npm start
2. API呼び出しの実装:AxiosなどのHTTPクライアントライブラリを使って、FastAPIのエンドポイントにリクエストを送信します。
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [items, setItems] = useState([]); useEffect(() => { axios.get('http://localhost:8000/items') .then(response => { setItems(response.data); }) .catch(error => { console.error("There was an error fetching the data!", error); }); }, []); return ( <div> <h1>Items</h1> {items.map((item, index) => ( <div key={index}> <h2>{item.name}</h2> <p>{item.description}</p> </div> ))} </div> ); } export default App;
3. UIの構築:Reactのコンポーネントを使って、フロントエンドのUIを構築します。
以上の手順により、ReactとFastAPIを連携させたフルスタックのWebアプリケーションが完成します。
このアプローチにより、モダンで効率的な開発が可能となります。
FastAPIとReactを使ったアプリケーション開発:ベストプラクティスと例
FastAPIとReactを組み合わせたアプリケーション開発は、シンプルかつ高効率なプロジェクトを構築するための強力な方法です。
この記事では、FastAPIとReactを使ったアプリケーション開発のベストプラクティスと、具体的な例について詳しく説明します。
まず、プロジェクトのセットアップから始め、アプリケーションの各部分がどのように連携するかを示します。
さらに、セキュリティ、パフォーマンス、スケーラビリティなどの重要な要素についても解説します。
以下に、FastAPIとReactを使ったプロジェクトの具体例を示します。
FastAPIのセットアップ
from fastapi import FastAPI, HTTPException from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str description: str = None price: float tax: float = None items = {} @app.post("/items/") def create_item(item_id: int, item: Item): if item_id in items: raise HTTPException(status_code=400, detail="Item already exists") items[item_id] = item return item @app.get("/items/{item_id}") def read_item(item_id: int): if item_id not in items: raise HTTPException(status_code=404, detail="Item not found") return items[item_id]
ReactのセットアップとAPI呼び出し
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [items, setItems] = useState([]); const [newItem, setNewItem] = useState({ name: '', description: '', price: 0, tax: 0 }); useEffect(() => { axios.get('http://localhost:8000/items') .then(response => { setItems(response.data); }) .catch(error => { console.error("There was an error fetching the data!", error); }); }, []); const addItem = () => { axios.post('http://localhost:8000/items', newItem) .then(response => { setItems([...items, response.data]); setNewItem({ name: '', description: '', price: 0, tax: 0 }); }) .catch(error => { console.error("There was an error adding the item!", error); }); }; return ( <div> <h1>Items</h1> <div> <input type="text" placeholder="Name" value={newItem.name} onChange={e => setNewItem({ ...newItem, name: e.target.value })} /> <input type="text" placeholder="Description" value={newItem.description} onChange={e => setNewItem({ ...newItem, description: e.target.value })} /> <input type="number" placeholder="Price" value={newItem.price} onChange={e => setNewItem({ ...newItem, price: parseFloat(e.target.value) })} /> <input type="number" placeholder="Tax" value={newItem.tax} onChange={e => setNewItem({ ...newItem, tax: parseFloat(e.target.value) })} /> <button onClick={addItem}>Add Item</button> </div> {items.map((item, index) => ( <div key={index}> <h2>{item.name}</h2> <p>{item.description}</p> <p>Price: {item.price}</p> <p>Tax: {item.tax}</p> </div> ))} </div> ); } export default App;
開発環境のセットアップとベストプラクティス
FastAPIとReactを使った開発では、開発環境のセットアップが重要です。
以下の手順に従って、効率的な開発環境を構築しましょう。
1. 仮想環境の設定:Pythonの仮想環境を作成し、必要なパッケージをインストールします。
python -m venv venv source venv/bin/activate pip install fastapi uvicorn
2. フロントエンドプロジェクトの作成:Create React Appを使用して、Reactプロジェクトを作成します。
npx create-react-app my-app cd my-app npm start
3. 依存関係の管理:必要なパッケージをインストールし、依存関係を管理します。
例えば、Axiosを使用してAPIと通信する場合は、以下のコマンドを実行します。
npm install axios
4. コードの整備:コードの可読性を高めるため、各モジュールやコンポーネントを適切に分割し、コメントを追加します。
また、LintやPrettierなどのツールを使ってコードスタイルを統一します。
FastAPIとReactの統合テストとデバッグ
FastAPIとReactを統合したアプリケーションのテストとデバッグは、アプリケーションの品質を確保するために重要です。
以下の手順でテストとデバッグを行います。
1. ユニットテストの実装:FastAPIでは、Pytestを使用してユニットテストを実装します。
from fastapi.testclient import TestClient from main import app client = TestClient(app) def test_read_root(): response = client.get("/") assert response.status_code == 200 assert response.json() == {"message": "Hello World"}
2. フロントエンドのテスト:Reactでは、JestやReact Testing Libraryを使用してコンポーネントのテストを行います。
import { render, screen } from '@testing-library/react'; import App from './App'; test('renders items', () => { render(<App />); const linkElement = screen.getByText(/Items/i); expect(linkElement).toBeInTheDocument(); });
3. 統合テストの実施:フロントエンドとバックエンドが正しく連携していることを確認するため、統合テストを実施します。
Mockデータやテスト用のデータベースを使用して、実際のデータと同様の環境を再現します。
4. デバッグの手法:デバッグツールを使用して、フロントエンドとバックエンドの両方のバグを特定し修正します。
ブラウザの開発者ツールや、Pythonのデバッガ(例:PDB)を活用します。
セキュリティとパフォーマンスの最適化
FastAPIとReactを使用したアプリケーションのセキュリティとパフォーマンスを最適化するためのベストプラクティスを紹介します。
1. 認証と認可:JWT(JSON Web Token)を使用して、ユーザー認証と認可を実装します。
FastAPIのセキュリティ機能を活用し、安全なAPIエンドポイントを構築します。
from fastapi import Depends, FastAPI, HTTPException, status from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm from jose import JWTError, jwt app = FastAPI() oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") @app.post("/token") async def login(form_data: OAuth2PasswordRequestForm = Depends()): # ユーザー認証ロジック pass @app.get("/users/me") async def read_users_me(token: str = Depends(oauth2_scheme)): # トークン検証ロジック pass
2. CORSの設定:フロントエンドとバックエンド間でのCORS(クロスオリジンリソースシェアリング)を適切に設定し、セキュリティを強化します。
from fastapi.middleware.cors import CORSMiddleware app.add_middleware( CORSMiddleware, allow_origins=["*"], allow_credentials=True, allow_methods=["*"], allow_headers=["*"], )
3. パフォーマンスの最適化:キャッシュの使用、データベースクエリの最適化、非同期処理の導入など、パフォーマンスを向上させるための手法を適用します。
4. スケーラビリティの確保:コンテナ化(Dockerの使用)、クラウドデプロイメント
(例:AWS、GCP)を通じて、アプリケーションのスケーラビリティを確保します。
以上のベストプラクティスを適用することで、FastAPIとReactを使用したアプリケーションの品質と効率を大幅に向上させることができます。
FastAPIとReactの連携による効率的なWebアプリケーション構築法
FastAPIとReactの連携により、効率的なWebアプリケーションを構築することが可能です。
この組み合わせは、モダンなフロントエンドと高速なバックエンドを提供し、全体的なパフォーマンスとユーザー体験を向上させます。
この記事では、FastAPIとReactを連携させるための詳細なステップとベストプラクティスについて説明します。
FastAPIの設定と基本的なAPIエンドポイントの作成
まず、FastAPIを設定し、基本的なAPIエンドポイントを作成します。
from fastapi import FastAPI, HTTPException from pydantic import BaseModel app = FastAPI() class User(BaseModel): username: str email: str users = [] @app.post("/users/") def create_user(user: User): users.append(user) return user @app.get("/users/") def read_users(): return users @app.get("/users/{username}") def read_user(username: str): for user in users: if user.username == username: return user raise HTTPException(status_code=404, detail="User not found")
Reactアプリケーションの構築とAPI呼び出し
次に、Reactアプリケーションを構築し、FastAPIのエンドポイントにリクエストを送信します。
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [users, setUsers] = useState([]); const [username, setUsername] = useState(''); const [email, setEmail] = useState(''); useEffect(() => { axios.get('http://localhost:8000/users/') .then(response => { setUsers(response.data); }) .catch(error => { console.error("There was an error fetching the users!", error); }); }, []); const addUser = () => { axios.post('http://localhost:8000/users/', { username, email }) .then(response => { setUsers([...users, response.data]); setUsername(''); setEmail(''); }) .catch(error => { console.error("There was an error adding the user!", error); }); }; return ( <div> <h1>Users</h1> <div> <input type="text" placeholder="Username" value={username} onChange={e => setUsername(e.target.value)} /> <input type="email" placeholder="Email" value={email} onChange={e => setEmail(e.target.value)} /> <button onClick={addUser}>Add User</button> </div> {users.map((user, index) => ( <div key={index}> <h2>{user.username}</h2> <p>{user.email}</p> </div> ))} </div> ); } export default App;
アプリケーション構築のステップバイステップガイド
FastAPIとReactを使ったアプリケーション構築には、以下のステップがあります。
1. FastAPIのセットアップ:Pythonの仮想環境を作成し、FastAPIとUvicornをインストールします。
python -m venv venv source venv/bin/activate pip install fastapi uvicorn
2. Reactのセットアップ:Create React Appを使って、Reactプロジェクトを作成します。
npx create-react-app my-app cd my-app npm start
3. APIエンドポイントの作成:FastAPIで必要なAPIエンドポイントを定義します。
4. フロントエンドの構築:ReactでUIコンポーネントを作成し、Axiosなどを使ってAPIと通信します。
5. アプリケーションのデプロイ:Dockerを使用してアプリケーションをコンテナ化し、クラウドプラットフォームにデプロイします。
FastAPIとReactのデプロイメントとスケーラビリティ
アプリケーションを本番環境にデプロイする際、スケーラビリティとパフォーマンスを考慮することが重要です。
以下の手順で、FastAPIとReactのアプリケーションをDockerでコンテナ化し、クラウドにデプロイします。
1. Dockerfileの作成:FastAPIとReactのそれぞれのためにDockerfileを作成します。
FastAPIのDockerfile
FROM python:3.9 WORKDIR /app COPY requirements.txt . RUN pip install -r requirements.txt COPY . . CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "80"]
ReactのDockerfile
FROM node:14 WORKDIR /app COPY package.json . RUN npm install COPY . . RUN npm run build CMD ["npx", "serve", "-s", "build"]
2. Docker Composeの設定:Docker Composeを使って、FastAPIとReactのサービスを設定します。
version: '3' services: fastapi: build: ./fastapi ports: - "8000:80" react: build: ./react ports: - "3000:5000"
3. デプロイ:Docker Composeを使ってアプリケーションを起動します。
docker-compose up --build
これにより、FastAPIとReactの連携アプリケーションがコンテナ化され、スケーラブルな環境でデプロイされます。
ユーザー認証とセッション管理
アプリケーションのセキュリティを強化するために、ユーザー認証とセッション管理を実装します。
1. JWTを使用した認証:JWT(JSON Web Token)を使って、ユーザー認証を実装します。
FastAPIの認証ロジック
from fastapi import Depends, FastAPI, HTTPException, status from fastapi.security import OAuth2PasswordBearer, OAuth2PasswordRequestForm from jose import JWTError, jwt from datetime import datetime, timedelta SECRET_KEY = "your_secret_key" ALGORITHM = "HS256" ACCESS_TOKEN_EXPIRE_MINUTES = 30 app = FastAPI() oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") def create_access_token(data: dict): to_encode = data.copy() expire = datetime.utcnow() + timedelta(minutes=ACCESS_TOKEN_EXPIRE_MINUTES) to_encode.update({"exp": expire}) encoded_jwt = jwt.encode(to_encode, SECRET_KEY, algorithm=ALGORITHM) return encoded_jwt @app.post("/token") async def login(form_data: OAuth2PasswordRequestForm = Depends()): # ユーザー認証ロジック access_token = create_access_token(data={"sub": form_data.username}) return {"access_token": access_token, "token_type": "bearer"} @app.get("/users/me") async def read_users_me(token: str = Depends(oauth2_scheme)): # トークン検証ロジック pass
2. Reactでのトークン管理:トークンをローカルストレージに保存し、APIリクエストに含めます。
import React, { useState } from 'react'; import axios from 'axios'; function Login() { const [username, setUsername] = useState(''); const [password, setPassword] = useState(''); const handleSubmit = () => { axios.post('http://localhost:8000/token', { username, password }) .then(response => { localStorage.setItem('token', response.data.access_token); }) .catch(error => { console.error("There was an error logging in!", error); }); }; return ( <div> <input type="text" placeholder="Username" value={username} onChange={e => setUsername(e.target.value)} /> <input type="password" placeholder="Password" value={password} onChange={e => setPassword(e.target.value)} /> <button onClick={handleSubmit}>Login</button> </div> ); } export default Login;
これにより、FastAPIとReactを連携させたアプリケーションの構築、デプロイ、セキュリティの強化が実現します。
上述の手法を適用することで、スケーラブルでセキュアなWebアプリケーションを効率的に開発することができます。
Dockerを用いたFastAPIとReactのコンテナ化とデプロイ方法
Dockerを使用することで、FastAPIとReactアプリケーションを簡単にコンテナ化し、さまざまな環境で一貫してデプロイすることができます。
この記事では、FastAPIとReactをDockerでコンテナ化する手順と、その後のデプロイ方法について詳しく解説します。
Dockerの基本設定とコンテナ化のメリット
Dockerは、アプリケーションとその依存関係を一つのコンテナにまとめることで、環境の違いによる問題を回避し、一貫した動作を保証します。
これにより、開発環境、本番環境、および他の環境間での移行が容易になります。
以下に、FastAPIとReactの基本的なDocker設定を示します。
FastAPIのDockerfile
FastAPIアプリケーションのためのDockerfileを作成します。
このファイルには、アプリケーションの依存関係をインストールし、FastAPIアプリケーションを実行するためのコマンドが含まれています。
FROM python:3.9 WORKDIR /app COPY requirements.txt . RUN pip install -r requirements.txt COPY . . CMD ["uvicorn", "main:app", "--host", "0.0.0.0", "--port", "80"]
ReactのDockerfile
次に、ReactアプリケーションのためのDockerfileを作成します。
このファイルには、Reactアプリケーションをビルドし、静的ファイルとして提供するための設定が含まれています。
FROM node:14 WORKDIR /app COPY package.json . RUN npm install COPY . . RUN npm run build CMD ["npx", "serve", "-s", "build"]
Docker Composeの設定
Docker Composeを使用して、FastAPIとReactの両方のサービスを一緒に起動します。
以下は、Docker Composeの設定ファイル(docker-compose.yml)の例です。
version: '3' services: fastapi: build: ./fastapi ports: - "8000:80" react: build: ./react ports: - "3000:5000"
アプリケーションのデプロイ手順
Docker Composeを使用してアプリケーションを起動し、デプロイします。
以下のコマンドを実行することで、FastAPIとReactの両方のコンテナが起動します。
docker-compose up --build
このコマンドにより、FastAPIアプリケーションはポート8000で、Reactアプリケーションはポート3000でそれぞれ起動します。
これにより、開発者はローカル環境で両方のサービスを簡単にテストし、開発を進めることができます。
クラウド環境へのデプロイ
DockerイメージをビルドしてDocker Hubにプッシュし、クラウド環境(例えば、AWS、GCP、Azure)にデプロイすることができます。
以下は、AWSにデプロイするための基本的な手順です。
1. Dockerイメージのビルド:
docker build -t my-fastapi-app ./fastapi docker build -t my-react-app ./react
2. Docker Hubにプッシュ:
docker tag my-fastapi-app mydockerhubusername/my-fastapi-app docker tag my-react-app mydockerhubusername/my-react-app docker push mydockerhubusername/my-fastapi-app docker push mydockerhubusername/my-react-app
3. クラウドプロバイダでの設定:
AWS ECSやEKS、GCPのGKE、AzureのAKSなどのクラウドプロバイダのサービスを使用して、コンテナ化されたアプリケーションをデプロイします。
以上の手順により、FastAPIとReactをコンテナ化し、異なる環境でのデプロイが簡単になります。
これにより、アプリケーションの可搬性が向上し、どの環境でも一貫した動作が保証されます。
FastAPIをフロントエンドとして使用するためのステップバイステップガイド
FastAPIは通常バックエンドとして使用されますが、フロントエンド機能を提供することもできます。
特に、APIエンドポイントを提供しながら、静的ファイル(HTML、CSS、JavaScriptなど)をサーブすることで、単一のアプリケーションとして機能させることができます。
この記事では、FastAPIをフロントエンドとして使用する方法についてステップバイステップで解説します。
FastAPIのセットアップと静的ファイルのサービング
まず、FastAPIをセットアップし、静的ファイルを提供するための基本設定を行います。
必要なパッケージのインストール
FastAPIと依存関係をインストールします。
pip install fastapi uvicorn jinja2 aiofiles
ディレクトリ構造の設定
静的ファイルとテンプレートファイルを管理するためのディレクトリを設定します。
/app ├── main.py ├── static │ ├── css │ └── js └── templates └── index.html
FastAPIのコード
静的ファイルとテンプレートを提供するためのFastAPIのコードを記述します。
from fastapi import FastAPI from fastapi.staticfiles import StaticFiles from fastapi.templating import Jinja2Templates from starlette.requests import Request app = FastAPI() app.mount("/static", StaticFiles(directory="static"), name="static") templates = Jinja2Templates(directory="templates") @app.get("/") async def read_root(request: Request): return templates.TemplateResponse("index.html", {"request": request})
HTMLテンプレートの作成
次に、テンプレートファイル(index.html)を作成します。
このファイルには、基本的なHTML構造と、必要に応じてCSSやJavaScriptファイルのリンクが含まれます。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>FastAPI Frontend</title> <link rel="stylesheet" href="/static/css/style.css"> </head> <body> <h1>Hello, FastAPI with Frontend!</h1> <script src="/static/js/main.js"></script> </body> </html>
静的ファイルの作成
続いて、静的ファイル(CSSおよびJavaScriptファイル)を作成します。
style.css
body { font-family: Arial, sans-serif; background-color: #f0f0f0; margin: 0; padding: 0; display: flex; justify-content: center; align-items: center; height: 100vh; } h1 { color: #333; }
main.js
console.log("FastAPI with frontend is working!");
アプリケーションの実行
FastAPIアプリケーションを実行して、フロントエンドを提供します。
uvicorn main:app --reload
これで、FastAPIアプリケーションが静的ファイルとHTMLテンプレートを提供するようになります。
ブラウザで`http://localhost:8000`にアクセスすると、設定したフロントエンドが表示されます。
APIエンドポイントの追加
フロントエンドに加えて、FastAPIを使用してAPIエンドポイントを追加することもできます。
以下の例では、基本的なCRUD操作を提供するエンドポイントを実装します。
FastAPIのコードの拡張
from fastapi import FastAPI, HTTPException from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str description: str = None price: float tax: float = None items = {} @app.post("/items/") async def create_item(item_id: int, item: Item): if item_id in items: raise HTTPException(status_code=400, detail="Item already exists") items[item_id] = item return item @app.get("/items/{item_id}") async def read_item(item_id: int): if item_id not in items: raise HTTPException(status_code=404, detail="Item not found") return items[item_id]
これにより、フロントエンドとバックエンドが統合されたアプリケーションが完成します。
FastAPIを使用して静的ファイルを提供し、APIエンドポイントを追加することで、単一のアプリケーションとして機能させることができます。
これにより、開発者は一貫した環境で効率的に開発を進めることができます。
FastAPIとReactの併用による高速なWebサーバー構築の実践
FastAPIとReactを組み合わせることで、高速かつ効率的なWebサーバーを構築することができます。
この記事では、FastAPIとReactを使用したWebサーバーの構築手順と、それによるメリットについて詳しく説明します。
FastAPIとReactの基本的なセットアップ
まず、FastAPIとReactのプロジェクトをセットアップします。
以下の手順に従って、環境を構築します。
FastAPIのセットアップ
python -m venv venv source venv/bin/activate pip install fastapi uvicorn
Reactのセットアップ
npx create-react-app my-app cd my-app npm start
APIエンドポイントの作成とテスト
FastAPIで基本的なAPIエンドポイントを作成し、Reactアプリケーションからテストします。
FastAPIのコード
from fastapi import FastAPI, HTTPException from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str description: str = None price: float tax: float = None items = {} @app.post("/items/") async def create_item(item_id: int, item: Item): if item_id in items: raise HTTPException(status_code=400, detail="Item already exists") items[item_id] = item return item @app.get("/items/{item_id}") async def read_item(item_id: int): if item_id not in items: raise HTTPException(status_code=404, detail="Item not found") return items[item_id]
Reactのコード
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [items, setItems] = useState([]); const [newItem, setNewItem] = useState({ name: '', description: '', price: 0, tax: 0 }); useEffect(() => { axios.get('http://localhost:8000/items') .then(response => { setItems(response.data); }) .catch(error => { console.error("There was an error fetching the data!", error); }); }, []); const addItem = () => { axios.post('http://localhost:8000/items', newItem) .then(response => { setItems([...items, response.data]); setNewItem({ name: '', description: '', price: 0, tax: 0 }); }) .catch(error => { console.error("There was an error adding the item!", error); }); }; return ( <div> <h1>Items</h1> <div> <input type="text" placeholder="Name" value={newItem.name} onChange={e => setNewItem({ ...newItem, name: e.target.value })} /> <input type="text" placeholder="Description" value={newItem.description} onChange={e => setNewItem({ ...newItem, description: e.target.value })} /> <input type="number" placeholder="Price" value={newItem.price} onChange={e => setNewItem({ ...newItem, price: parseFloat(e.target.value) })} /> <input type="number" placeholder="Tax" value={newItem.tax} onChange={e => setNewItem({ ...newItem, tax: parseFloat(e.target.value) })} /> <button onClick={addItem}>Add Item</button> </div> {items.map((item, index) => ( <div key={index}> <h2>{item.name}</h2> <p>{item.description}</p> <p>Price: {item.price}</p> <p>Tax: {item.tax}</p> </div> ))} </div> ); } export default App;
パフォーマンス最
適化の手法
FastAPIとReactを使用したアプリケーションのパフォーマンスを最適化するための手法を紹介します。
1. 非同期処理の利用:FastAPIは非同期処理をサポートしており、大量のリクエストを効率的に処理することができます。
非同期関数(`async def`)を使用して、I/O操作を非同期に行います。
2. キャッシュの活用:APIレスポンスのキャッシュを利用することで、頻繁にアクセスされるデータの取得を高速化します。
Redisなどのキャッシュストアを使用して、データをキャッシュします。
3. CDNの利用:静的ファイル(CSS、JavaScript、画像など)をCDN(Content Delivery Network)で配信することで、ロード時間を短縮します。
CDNを使用することで、ユーザーの地理的な位置に関係なく高速なコンテンツ配信が可能になります。
4. コードの最適化:Reactのコードを最適化し、不要なレンダリングを避けるために、React.memoやuseMemo、useCallbackなどのフックを活用します。
また、Reactのビルド時にTree Shakingを有効にすることで、未使用のコードを削除します。
5. データベースの最適化:データベースクエリの最適化やインデックスの追加など、データベースのパフォーマンスを向上させるための手法を適用します。
SQLAlchemyやTortoise ORMなどのORMを使用する場合、効率的なクエリを作成することが重要です。
運用とモニタリング
アプリケーションを本番環境で運用する際には、継続的なモニタリングとメンテナンスが重要です。
以下のツールと手法を使用して、アプリケーションのパフォーマンスと信頼性を維持します。
1. ログ管理:ロギングを設定して、アプリケーションの動作状況を記録します。
FastAPIでは、標準のloggingモジュールを使用してログを記録します。
import logging logging.basicConfig(level=logging.INFO) logger = logging.getLogger(__name__) @app.get("/") async def read_root(): logger.info("Root endpoint accessed") return {"message": "Hello World"}
2. パフォーマンスモニタリング:New Relic、Datadog、Prometheusなどのパフォーマンスモニタリングツールを使用して、アプリケーションのパフォーマンスを監視します。
これにより、パフォーマンスのボトルネックを特定し、適切な対応を取ることができます。
3. エラートラッキング:Sentryなどのエラートラッキングツールを使用して、アプリケーションのエラーをリアルタイムで検出し、分析します。
これにより、エラーの影響を最小限に抑えることができます。
4. スケーリング:アプリケーションの負荷に応じて、自動スケーリングを設定します。
AWS EC2 Auto Scaling、Kubernetesなどのツールを使用して、アプリケーションを自動的にスケールアップまたはスケールダウンします。
以上の手法を適用することで、FastAPIとReactを使用した高速で信頼性の高いWebサーバーを構築し、効率的に運用することができます。
これにより、ユーザーに対して一貫した高性能なサービスを提供し続けることが可能となります。