SpringのDIの仕組みとは?基本概念とその重要性について解説
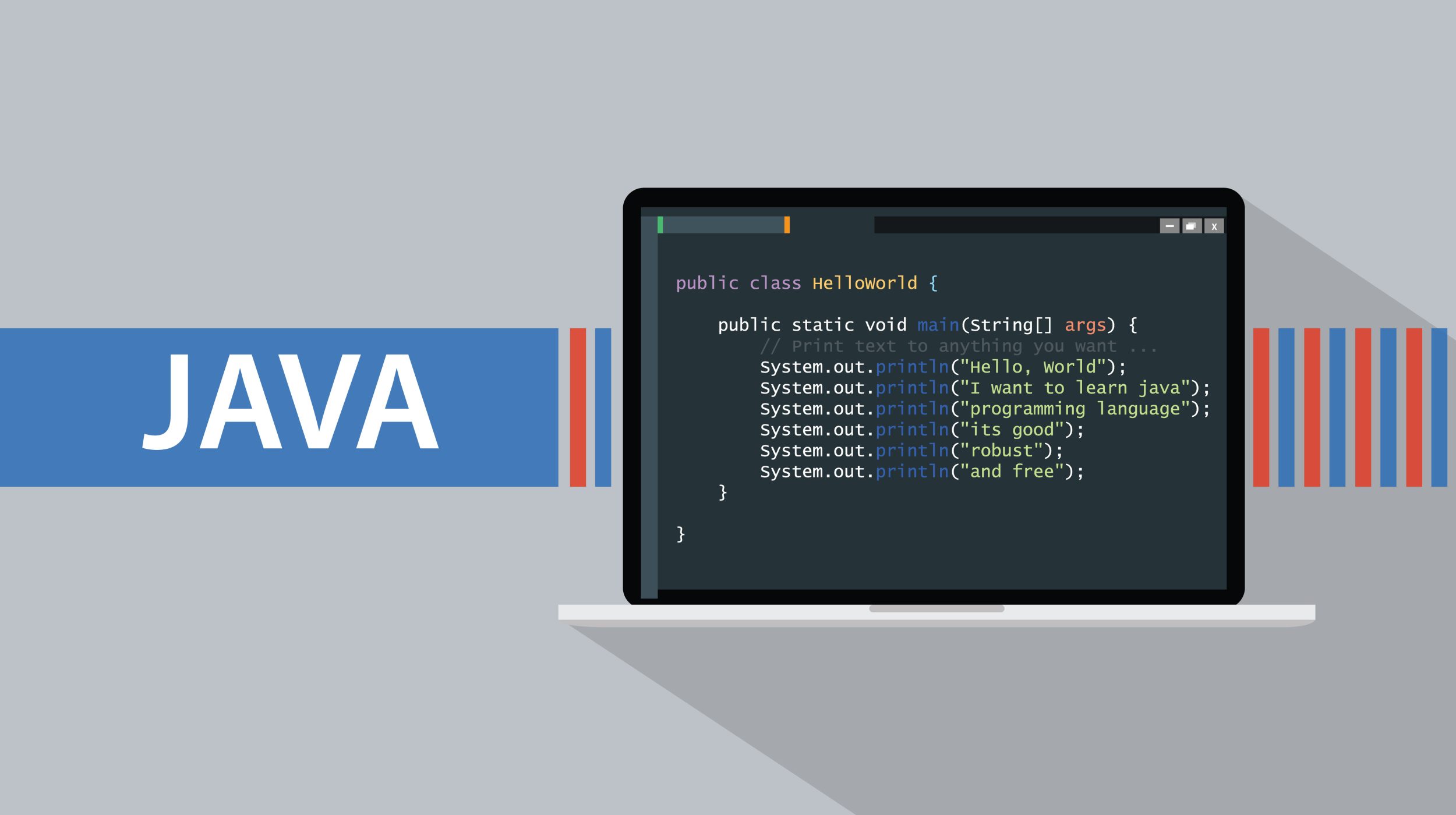
目次
SpringのDIの仕組みとは?基本概念とその重要性について解説
Springフレームワークの一つの柱となるDI(Dependency Injection)は、ソフトウェア開発において依存関係の管理を効率化するための重要な技術です。
DIの基本概念は、オブジェクトの生成やその依存関係を外部から注入することにより、オブジェクト同士の結びつきを緩やかにすることです。
このアプローチにより、モジュール間の結合度が低くなり、保守性や再利用性が向上します。
DIとは何か?その基本概念と歴史的背景
DIとは、依存性注入の略で、オブジェクトの生成や依存関係を外部から提供するデザインパターンです。
これにより、オブジェクト間の依存関係を宣言的に管理し、柔軟でテストしやすいコードを実現できます。
DIの歴史は、ソフトウェアアーキテクチャの進化と共にあり、特に2000年代初頭のエンタープライズアプリケーションの発展と共に注目されるようになりました。
SpringにおけるDIの役割と重要性
SpringフレームワークはDIを中核に据えており、これにより開発者は複雑な依存関係を簡単に管理できます。
SpringのDIは、アノテーションやXML設定ファイルを用いて実現され、コードの可読性とメンテナンス性が大幅に向上します。
DIを利用することで、アプリケーション全体の設計がシンプルかつ柔軟になり、開発効率が向上します。
SpringのDIの基本的な構成要素
SpringのDIは、主に以下の3つの構成要素から成り立っています。
1. Bean:Springコンテナが管理するオブジェクト。
2. ApplicationContext:Beanの生成や依存関係を管理するコンテナ。
3. アノテーション:依存関係を注入するために使用されるメタデータ。
以下は簡単なサンプルコードです。
import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.stereotype.Component; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } } public class Main { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); Client client = context.getBean(Client.class); client.doSomething(); } }
DIを用いることのメリットとデメリット
DIを利用することで得られる主なメリットは以下の通りです。
1. モジュール間の結合度が低くなるため、保守性が向上する。
2. 依存関係が明確になるため、コードの可読性が向上する。
3. テストが容易になるため、品質が向上する。
一方で、DIには以下のようなデメリットもあります。
1. 初期設定が複雑になることがある。
2. DIコンテナの理解に時間がかかる。
3. 過剰なDIの使用は、かえってコードの可読性を低下させる可能性がある。
以上のように、DIは効果的に使用することで大きなメリットをもたらしますが、適切に設計することが重要です。
Spring BootのDIコンテナの概要と基本的な実装方法
Spring Bootは、迅速なアプリケーション開発を可能にするためのフレームワークで、その中心にDIコンテナの概念があります。
DIコンテナは、アプリケーションのライフサイクルを管理し、依存関係を自動的に注入するための仕組みです。
これにより、開発者は依存関係の設定や管理に関する複雑さを軽減し、よりビジネスロジックに集中することができます。
Spring Bootは、アノテーションやJavaベースの設定を用いて簡単にDIコンテナを設定することができます。
DIコンテナとは何か?その基本概念と役割
DIコンテナは、依存性注入を実現するためのコンポーネントで、アプリケーション内のオブジェクトの生成やその依存関係を管理します。
DIコンテナの主な役割は、オブジェクト間の結びつきを外部から注入することで、オブジェクトの独立性を保ち、再利用性やテストの容易性を向上させることです。
これにより、モジュール間の結合度を低減し、ソフトウェアの保守性と拡張性を高めます。
Spring BootでのDIコンテナの設定方法と基本的な使い方
Spring BootでのDIコンテナの設定は非常にシンプルです。
アノテーションを用いることで、自動的に依存関係を注入することができます。
以下の例は、Spring BootアプリケーションでのDIコンテナの設定方法を示しています。
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; @SpringBootApplication @ComponentScan(basePackages = "com.example") public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } @Bean public Service service() { return new Service(); } @Bean public Client client(Service service) { return new Client(service); } }
このコードは、`@SpringBootApplication`アノテーションを使用してSpring Bootアプリケーションを構成し、`@Bean`アノテーションを使用してDIコンテナに登録するオブジェクトを定義しています。
アノテーションを用いたDIの実装例
Spring Bootでは、アノテーションを使用することで簡単に依存関係を注入することができます。
以下に、アノテーションを用いたDIの実装例を示します。
import org.springframework.stereotype.Component; import org.springframework.beans.factory.annotation.Autowired; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; @Autowired public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } }
この例では、`@Component`アノテーションを使用して`Service`および`Client`クラスをSpringコンテナに登録し、`@Autowired`アノテーションを使用して`Client`クラスの`Service`依存関係を注入しています。
XML設定ファイルを用いたDIの実装例
Spring Bootでは、アノテーションに加えてXML設定ファイルを使用してDIを設定することも可能です。
以下は、その実装例です。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="service" class="com.example.Service"/> <bean id="client" class="com.example.Client"> <constructor-arg ref="service"/> </bean> </beans>
このXMLファイルでは、`service`および`client`というIDを持つBeanを定義し、`client`のコンストラクタ引数として`service`を注入しています。
DIコンテナを活用したテスト手法とベストプラクティス
DIコンテナを活用することで、テストの容易性が向上します。
モックオブジェクトを使用することで、依存関係を注入してテストを行うことができます。
以下に、JUnitとMockitoを用いたテストの例を示します。
import static org.mockito.Mockito.*; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.boot.test.mock.mockito.MockBean; @SpringBootTest public class ClientTest { @Autowired private Client client; @MockBean private Service service; @Test public void testDoSomething() { doNothing().when(service).serve(); client.doSomething(); verify(service, times(1)).serve(); } }
このテストでは、`@MockBean`アノテーションを使用して`Service`のモックを作成し、`Client`の依存関係として注入しています。
これにより、`Client`のメソッドをテストし、`Service`が正しく呼び出されるかを確認できます
以上のように、Spring BootのDIコンテナを利用することで、依存関係の管理が容易になり、開発やテストが効率化されます。
DI(Dependency Injection)とは何か?その概念と利点を理解する
DI(Dependency Injection)は、ソフトウェアデザインパターンの一つで、オブジェクトが自ら依存するオブジェクトを生成するのではなく、外部から注入されるという仕組みです。
これにより、コードの柔軟性や再利用性が高まり、特に大規模なアプリケーションにおいてはその効果が顕著に現れます。
DIの主な利点としては、コードのモジュール化が進むこと、テストが容易になること、依存関係が明示的になることなどが挙げられます。
DIの基本概念とその重要性
DIの基本概念は、オブジェクトが他のオブジェクトに依存する場合、その依存関係を外部から提供することです。
これにより、オブジェクト間の結合度を低減し、個々のコンポーネントが独立して開発・テスト・デプロイできるようになります。
DIの重要性は、特にアプリケーションが大規模化するほど増していきます。
以下に、DIの基本概念を示すシンプルな例を紹介します。
public class Service { public void serve() { System.out.println("Service is serving..."); } } public class Client { private Service service; // Constructor injection public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } } // Main class public class Main { public static void main(String[] args) { Service service = new Service(); Client client = new Client(service); client.doSomething(); } }
この例では、`Client`クラスが`Service`クラスに依存していることがわかりますが、その依存関係はコンストラクタを通じて外部から注入されています。
これにより、`Client`クラスは`Service`クラスの具体的な実装に依存しなくなり、テストや変更が容易になります。
DIの利点とその活用方法
DIを使用することで得られる主な利点は次の通りです。
1. 再利用性の向上:依存関係が外部から注入されるため、同じオブジェクトを異なるコンテキストで再利用できます。
2. テストの容易さ:依存関係を簡単にモックオブジェクトに置き換えられるため、ユニットテストが容易になります。
3. コードの柔軟性:依存関係を変更する際に、最小限のコード変更で済むため、メンテナンスが容易になります。
DIコンテナとは何か?その機能と役割について解説
DIコンテナは、オブジェクトの生成およびその依存関係を管理するためのフレームワークです。
SpringのDIコンテナは、アプリケーションコンテキストと呼ばれ、Beanのライフサイクル管理や依存関係の解決を自動的に行います。
以下に、SpringのDIコンテナを使用した例を示します。
import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class AppConfig { @Bean public Service service() { return new Service(); } @Bean public Client client() { return new Client(service()); } } public class Main { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); Client client = context.getBean(Client.class); client.doSomething(); } }
この例では、`AppConfig`クラスにBean定義を行い、Springのアノテーションベースのコンフィギュレーションを使用してDIコンテナを設定しています。
これにより、オブジェクトの生成や依存関係の注入が自動化され、開発者はビジネスロジックに集中できます。
DIのコード例
DIの実装は、アノテーションやXML設定ファイルを使用して行います。
以下は、アノテーションを用いたDIのコード例です。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; @Autowired public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } } import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
このコードでは、`@Component`アノテーションを使用してSpringコンテナにBeanを登録し、`@Autowired`アノテーションを使用して依存関係を注入しています。
SpringのDIコンテナの設定方法と実践的な使い方
SpringのDIコンテナを利用することで、アプリケーションの構造をシンプルに保ち、依存関係を効率的に管理することができます。
Springでは、アノテーションやXML設定ファイルを使用して簡単にDIコンテナを設定することが可能です。
このセクションでは、DIコンテナの基本設定方法から、実践的な使い方までを詳しく解説します。
DIコンテナの基本設定
SpringのDIコンテナを設定するための基本的な方法は、アノテーションを使用することです。
以下は、Spring BootでDIコンテナを設定するためのシンプルな例です。
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; @SpringBootApplication @ComponentScan(basePackages = "com.example") public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } @Bean public Service service() { return new Service(); } @Bean public Client client(Service service) { return new Client(service); } }
この例では、`@SpringBootApplication`アノテーションを使用してSpring Bootアプリケーションを定義し、`@Bean`アノテーションを使用してDIコンテナにBeanを登録しています。
`ComponentScan`アノテーションは指定したパッケージをスキャンし、Bean定義を自動的に検出します。
Bean定義の方法とその活用例
Bean定義は、Springコンテナが管理するオブジェクトの設定を行う方法です。
アノテーションを使用したBean定義の例を以下に示します。
import org.springframework.stereotype.Component; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } }
この例では、`@Component`アノテーションを使用して`Service`および`Client`クラスをSpringコンテナに登録しています。
`Client`クラスは、コンストラクタを通じて`Service`のインスタンスを受け取ります。
アノテーションを用いた設定とその利点
アノテーションを使用することで、設定が簡素化され、コードの可読性が向上します。
`@Autowired`アノテーションを使用すると、Springが自動的に依存関係を解決し、注入します。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class Client { private final Service service; @Autowired public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } }
この例では、`@Autowired`アノテーションを使用して`Service`のインスタンスを`Client`クラスに注入しています。
これにより、依存関係の管理が自動化され、コードがシンプルになります。
XML設定ファイルを用いた設定方法
Springでは、アノテーションに加えてXML設定ファイルを使用してDIコンテナを設定することもできます。
以下にその例を示します。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="service" class="com.example.Service"/> <bean id="client" class="com.example.Client"> <constructor-arg ref="service"/> </bean> </beans>
このXML設定ファイルでは、`Service`および`Client`というIDを持つBeanを定義し、`Client`のコンストラクタ引数として`Service`を注入しています。
XML設定ファイルを使用することで、設定が外部化され、構成の変更が容易になります。
DIコンテナの高度な設定とカスタマイズ方法
SpringのDIコンテナは、様々なカスタマイズが可能です。
例えば、プロファイルを使用して環境ごとに異なる設定を適用することができます。
また、カスタムスコープを定義して、Beanのライフサイクルを柔軟に管理することも可能です。
import org.springframework.context.annotation.Scope; import org.springframework.stereotype.Component; @Component @Scope("prototype") public class PrototypeService { public void serve() { System.out.println("Prototype Service is serving..."); } }
この例では、`@Scope`アノテーションを使用して`PrototypeService`クラスのスコープをプロトタイプに設定しています。
これにより、SpringコンテナはこのBeanの新しいインスタンスを必要に応じて生成します。
以上のように、SpringのDIコンテナは、柔軟で強力な依存関係管理を提供し、アプリケーション開発を効率化します。
SpringにおけるBean定義とその役割について詳しく解説
SpringフレームワークにおけるBeanは、DIコンテナによって管理されるオブジェクトを指します。
Bean定義は、これらのオブジェクトの生成方法やライフサイクルを指定するための設定情報です。
Springでは、アノテーションやXML設定ファイルを用いてBean定義を行い、依存関係を効率的に管理することができます。
このセクションでは、Bean定義の基本概念から、具体的な定義方法、ライフサイクル管理までを詳しく解説します。
Bean定義の基本概念と役割
Bean定義は、Springコンテナがオブジェクトを管理するための基礎となる設定情報です。
これには、Beanのインスタンス化方法、依存関係の注入方法、Beanのライフサイクル管理に関する情報が含まれます。
Bean定義を通じて、開発者はアプリケーションの構成要素を明確にし、再利用性や保守性を高めることができます。
アノテーションを使用したBean定義の方法
Springでは、アノテーションを使用して簡単にBean定義を行うことができます。
以下は、`@Component`アノテーションを使用したBean定義の例です。
import org.springframework.stereotype.Component; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } }
この例では、`@Component`アノテーションを使用して、`Service`および`Client`クラスをSpringコンテナに登録しています。
これにより、Springはこれらのクラスを自動的にスキャンし、DIコンテナにBeanとして登録します。
XML設定ファイルを使用したBean定義の方法
XML設定ファイルを使用することで、アノテーションを使わずにBean定義を行うことも可能です。
以下は、XML設定ファイルを使用したBean定義の例です。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="service" class="com.example.Service"/> <bean id="client" class="com.example.Client"> <constructor-arg ref="service"/> </bean> </beans>
このXMLファイルでは、`service`および`client`というIDを持つBeanを定義し、`client`のコンストラクタ引数として`service`を注入しています。
XML設定ファイルを使用することで、設定情報を外部化し、管理が容易になります。
Beanのライフサイクルとスコープの設定方法
Springでは、Beanのライフサイクルやスコープを設定することができます。
ライフサイクルには、Beanの生成から破棄までの一連のプロセスが含まれ、スコープはBeanのインスタンスがどのように管理されるかを指定します。
以下は、スコープを設定する例です。
import org.springframework.context.annotation.Scope; import org.springframework.stereotype.Component; @Component @Scope("prototype") public class PrototypeService { public void serve() { System.out.println("Prototype Service is serving..."); } }
この例では、`@Scope`アノテーションを使用して`PrototypeService`クラスのスコープをプロトタイプに設定しています。
これにより、SpringコンテナはこのBeanの新しいインスタンスを必要に応じて生成します。
カスタムBean定義とその応用例
カスタムBean定義を作成することで、特定の要件に応じた柔軟なBean管理が可能になります。
以下は、カスタム初期化メソッドおよび破棄メソッドを指定する例です。
import javax.annotation.PostConstruct; import javax.annotation.PreDestroy; import org.springframework.stereotype.Component; @Component public class CustomService { @PostConstruct public void init() { System.out.println("CustomService is initialized"); } @PreDestroy public void destroy() { System.out.println("CustomService is destroyed"); } public void serve() { System.out.println("CustomService is serving..."); } }
この例では、`@PostConstruct`アノテーションを使用して初期化メソッドを、`@PreDestroy`アノテーションを使用して破棄メソッドを定義しています。
これにより、Beanのライフサイクルにカスタムロジックを追加することができます。
以上のように、SpringにおけるBean定義は、アプリケーションの構成要素を効率的に管理するための重要な手段です。
適切なBean定義を行うことで、ソフトウェアの保守性や拡張性が大幅に向上します。
DIコンテナとは何か?その機能と役割について解説
DIコンテナ(Dependency Injection Container)は、ソフトウェアアプリケーションの依存関係を管理するためのフレームワークです。
SpringのDIコンテナは、アプリケーションコンテキストと呼ばれ、Beanの生成、依存関係の解決、ライフサイクルの管理を自動化します。
これにより、開発者は複雑な依存関係を明示的に管理する必要がなくなり、コードのモジュール化やテストの容易性が向上します。
DIコンテナの基本概念と役割
DIコンテナの基本概念は、オブジェクトの生成とその依存関係を管理することです。
DIコンテナは、依存関係を外部から注入することで、オブジェクト間の結合度を低減し、コードの再利用性と保守性を高めます。
これにより、開発者はビジネスロジックに集中し、アプリケーションの設計を柔軟かつ効率的に行うことができます。
以下は、SpringのDIコンテナを使用した簡単な例です。
import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class AppConfig { @Bean public Service service() { return new Service(); } @Bean public Client client() { return new Client(service()); } } public class Main { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); Client client = context.getBean(Client.class); client.doSomething(); } } class Service { public void serve() { System.out.println("Service is serving..."); } } class Client { private final Service service; public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } }
この例では、`AppConfig`クラスにBean定義を行い、`AnnotationConfigApplicationContext`を使用してアプリケーションコンテキストを作成しています。
これにより、`Client`クラスのインスタンスは、依存する`Service`クラスのインスタンスを自動的に注入されます。
DIコンテナのメリットとデメリット
DIコンテナを使用することには多くのメリットがありますが、いくつかのデメリットも存在します。
メリット:
1. 再利用性の向上: オブジェクト間の結合度が低いため、モジュールの再利用が容易になります。
2. テストの容易さ: 依存関係が外部から注入されるため、モックオブジェクトを使用したユニットテストが簡単になります。
3. 保守性の向上: 依存関係が明確になるため、コードの保守が容易になります。
デメリット:
1. 初期設定の複雑さ: DIコンテナの設定には学習コストがかかります。
2. パフォーマンスのオーバーヘッド: DIコンテナの使用により、アプリケーションの起動時にパフォーマンスのオーバーヘッドが発生することがあります。
3. 過剰な抽象化のリスク: DIを過剰に使用すると、コードが過度に抽象化され、理解しづらくなることがあります。
DIコンテナの設定と使用例
DIコンテナの設定は、アノテーションやXML設定ファイルを使用して行います。
以下に、XML設定ファイルを使用したDIコンテナの設定例を示します。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="service" class="com.example.Service"/> <bean id="client" class="com.example.Client"> <constructor-arg ref="service"/> </bean> </beans>
このXML設定ファイルでは、`service`および`client`というIDを持つBeanを定義し、`client`のコンストラクタ引数として`service`を注入しています。
XML設定ファイルを使用することで、設定が外部化され、構成の変更が容易になります。
DIコンテナの高度な設定とカスタマイズ方法
SpringのDIコンテナは、プロファイルを使用して環境ごとに異なる設定を適用することができます。
また、カスタムスコープを定義して、Beanのライフサイクルを柔軟に管理することも可能です。
以下に、カスタム初期化メソッドおよび破棄メソッドを指定する例を示します。
import javax.annotation.PostConstruct; import javax.annotation.PreDestroy; import org.springframework.stereotype.Component; @Component public class CustomService { @PostConstruct public void init() { System.out.println("CustomService is initialized"); } @PreDestroy public void destroy() { System.out.println("CustomService is destroyed"); } public void serve() { System.out.println("CustomService is serving..."); } }
この例では、`@PostConstruct`アノテーションを使用して初期化メソッドを、`@PreDestroy`アノテーションを使用して破棄メソッドを定義しています。
これにより、Beanのライフサイクルにカスタムロジックを追加することができます。
以上のように、DIコンテナを活用することで、アプリケーションの依存関係管理が効率化され、開発やテストが容易になります。
DIのコード例
DI(Dependency Injection)の実装例を通じて、実際のコードにおけるDIの適用方法を理解しましょう。
ここでは、基本的なDIのコード例から、アノテーションやXML設定ファイルを用いた高度なDIの実装方法、プロファイルを用いた環境ごとのDI設定、テスト環境におけるDIの利用方法とベストプラクティスについて解説します。
基本的なDIのコード例とその解説
DIの基本的な実装例として、以下のコードを見てみましょう。
この例では、`Service`クラスを`Client`クラスに注入します。
public class Service { public void serve() { System.out.println("Service is serving..."); } } public class Client { private final Service service; // Constructor injection public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } } // Main class public class Main { public static void main(String[] args) { Service service = new Service(); Client client = new Client(service); client.doSomething(); } }
このコードでは、`Client`クラスが`Service`クラスに依存していることがわかります。
依存関係は、`Client`クラスのコンストラクタを通じて外部から注入されています。
これにより、`Client`クラスは`Service`クラスの具体的な実装に依存しなくなり、テストや変更が容易になります。
アノテーションを用いた高度なDIのコード例
Springでは、アノテーションを使用することで、依存関係の注入をさらに簡素化できます。
以下は、`@Autowired`アノテーションを使用したDIの実装例です。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; @Autowired public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } } import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
このコードでは、`@Component`アノテーションを使用して`Service`および`Client`クラスをSpringコンテナに登録し、`@Autowired`アノテーションを使用して依存関係を注入しています。
これにより、Springが自動的に依存関係を解決し、注入します。
XML設定ファイルを用いたDIのコード例
Springでは、アノテーションに加えてXML設定ファイルを使用してDIを設定することも可能です。
以下は、その実装例です。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="service" class="com.example.Service"/> <bean id="client" class="com.example.Client"> <constructor-arg ref="service"/> </bean> </beans>
このXMLファイルでは、`service`および`client`というIDを持つBeanを定義し、`client`のコンストラクタ引数として`service`を注入しています。
XML設定ファイルを使用することで、設定が外部化され、構成の変更が容易になります。
プロファイルを用いた環境ごとのDI設定例
Springでは、プロファイルを使用して環境ごとに異なる設定を適用することができます。
以下は、プロファイルを用いたDI設定の例です。
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Profile; @Configuration public class AppConfig { @Bean @Profile("development") public Service devService() { return new DevService(); } @Bean @Profile("production") public Service prodService() { return new ProdService(); } }
この例では、`@Profile`アノテーションを使用して開発環境用と本番環境用のBeanを定義しています。
Springアプリケーションを起動する際に、特定のプロファイルをアクティブにすることで、環境ごとに異なる設定を適用することができます。
テスト環境におけるDIの利用方法とベストプラクティス
テスト環境でのDIの利用は、モックオブジェクトを使用することで大幅に簡素化されます。
以下に、JUnitとMockitoを用いたテストの例を示します。
import static org.mockito.Mockito.*; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.boot.test.mock.mockito.MockBean; @SpringBootTest public class ClientTest { @Autowired private Client client; @MockBean private Service service; @Test public void testDoSomething() { doNothing().when(service).serve(); client.doSomething(); verify(service, times(1)).serve(); } }
このテストでは、`@MockBean`アノテーションを使用して`Service`のモックを作成し、`Client`の依存関係として注入しています。
これにより、`Client`のメソッドをテストし、`Service`が正しく呼び出されるかを確認できます。
以上のように、DIを活用することで、依存関係の管理が効率化され、アプリケーションの開発やテストが容易になります。
SpringのDIコンテナを効果的に利用することで、ソフトウェアの保守性や再利用性を高めることができます。
Springを用いたDIのコード例と具体的な実装手順
Springフレームワークを用いたDIの実装は、アプリケーションの設計を柔軟かつ効率的にするための重要な手法です。
ここでは、具体的なコード例を通じて、DIの実装手順を詳細に解説します。
これには、基本的なアノテーションの使用方法から、設定ファイルを用いたアプローチまでを含みます。
基本的なDIの実装例
まず、基本的なDIの実装例を示します。
ここでは、Springのアノテーションを使用して依存関係を管理します。
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class Service { public void serve() { System.out.println("Service is serving..."); } } @Component public class Client { private final Service service; @Autowired public Client(Service service) { this.service = service; } public void doSomething() { service.serve(); } } import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class Application { public static void main(String[] args) { SpringApplication.run(Application.class, args); } }
この例では、`@Component`アノテーションを使用して`Service`および`Client`クラスをSpringコンテナに登録しています。
`@Autowired`アノテーションを使用することで、Springが自動的に`Client`クラスに`Service`クラスのインスタンスを注入します。
XML設定ファイルを用いたDIの実装例
Springでは、アノテーションに加えてXML設定ファイルを使用してDIを設定することも可能です。
以下は、その実装例です。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <bean id="service" class="com.example.Service"/> <bean id="client" class="com.example.Client"> <constructor-arg ref="service"/> </bean> </beans>
このXMLファイルでは、`service`および`client`というIDを持つBeanを定義し、`client`のコンストラクタ引数として`service`を注入しています。
XML設定ファイルを使用することで、設定が外部化され、構成の変更が容易になります。
プロファイルを用いた環境ごとのDI設定例
Springでは、プロファイルを使用して環境ごとに異なる設定を適用することができます。
以下は、プロファイルを用いたDI設定の例です。
import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Profile; @Configuration public class AppConfig { @Bean @Profile("development") public Service devService() { return new DevService(); } @Bean @Profile("production") public Service prodService() { return new ProdService(); } }
この例では、`@Profile`アノテーションを使用して開発環境用と本番環境用のBeanを定義しています。
Springアプリケーションを起動する際に、特定のプロファイルをアクティブにすることで、環境ごとに異なる設定を適用することができます。
テスト環境におけるDIの利用方法とベストプラクティス
テスト環境でのDIの利用は、モックオブジェクトを使用することで大幅に簡素化されます。
以下に、JUnitとMockitoを用いたテストの例を示します。
import static org.mockito.Mockito.*; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.boot.test.mock.mockito.MockBean; @SpringBootTest public class ClientTest { @Autowired private Client client; @MockBean private Service service; @Test public void testDoSomething() { doNothing().when(service).serve(); client.doSomething(); verify(service, times(1)).serve(); } }
このテストでは、`@MockBean`アノテーションを使用して`Service`のモックを作成し、`Client`の依存関係として注入しています。
これにより、`Client`のメソッドをテストし、`Service`が正しく呼び出されるかを確認できます。
高度なDIの設定とカスタマイズ
SpringのDIは非常に柔軟であり、カスタマイズ可能です。
例えば、プロトタイプスコープのBeanやカスタム初期化メソッド、破棄メソッドを使用することができます。
import org.springframework.context.annotation.Scope; import org.springframework.stereotype.Component; @Component @Scope("prototype") public class PrototypeService { public void serve() { System.out.println("Prototype Service is serving..."); } }
この例では、`@Scope`アノテーションを使用して`PrototypeService`クラスのスコープをプロトタイプに設定しています。
これにより、SpringコンテナはこのBeanの新しいインスタンスを必要に応じて生成します。
また、カスタム初期化メソッドおよび破棄メソッドを指定することで、Beanのライフサイクルにカスタムロジックを追加することも可能です。
import javax.annotation.PostConstruct; import javax.annotation.PreDestroy; import org.springframework.stereotype.Component; @Component public class CustomService { @PostConstruct public void init() { System.out.println("CustomService is initialized"); } @PreDestroy public void destroy() { System.out.println("CustomService is destroyed"); } public void serve() { System.out.println("CustomService is serving..."); } }
この例では、`@PostConstruct`アノテーションを使用して初期化メソッドを、`@PreDestroy`アノテーションを使用して破棄メソッドを定義しています。
これにより、Beanのライフサイクルにカスタムロジックを追加することができます。
以上のように、Springを用いたDIの実装は、依存関係の管理を効率化し、アプリケーションの設計を柔軟かつ効率的にします。
様々なアノテーションや設定ファイルを使用することで、複雑な依存関係も簡単に管理できるようになります。